Website Traffic Hackerrank Solution Sql Server
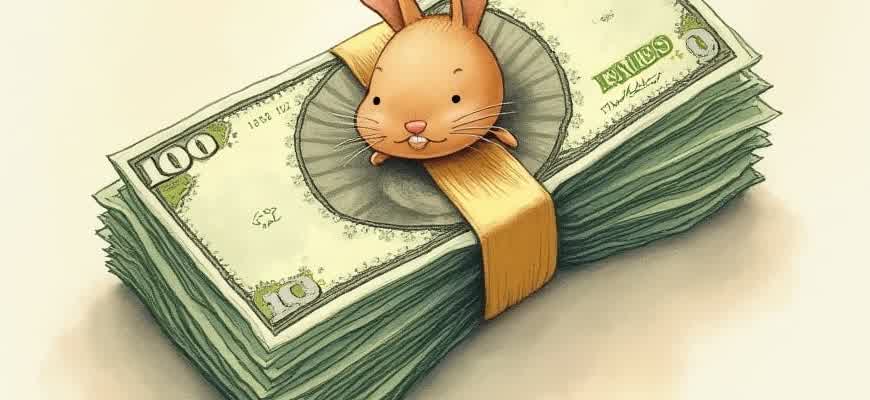
When dealing with large volumes of data related to website traffic, it is essential to utilize efficient database queries. SQL Server provides powerful tools for analyzing traffic patterns and extracting meaningful insights. Below are key techniques for optimizing traffic analysis.
- Use proper indexing to speed up query performance.
- Aggregate data into summary tables to avoid recalculating statistics for every query.
- Leverage JOIN operations to combine traffic data with user behavior metrics.
SQL queries can be optimized to identify trends and bottlenecks in the traffic data. The following steps outline how to structure a query that efficiently aggregates website visits:
- First, filter traffic data based on relevant time periods (e.g., daily, weekly, monthly).
- Next, apply GROUP BY to categorize data by user demographics or traffic source.
- Finally, apply ORDER BY to sort the results and reveal the most important trends.
Important: When dealing with large datasets, always ensure your queries are optimized to avoid long execution times.
The table below provides an example of a simple query structure used to aggregate website traffic data by source and date:
Source | Date | Visits |
---|---|---|
Organic Search | 2025-04-01 | 1200 |
Direct Traffic | 2025-04-01 | 800 |
Website Traffic Analysis Solution for SQL Server
When working with large sets of website traffic data in SQL Server, it is essential to efficiently aggregate and process user visits. A typical problem often involves identifying user visits and determining the most active times or pages. SQL queries must be optimized to handle these large volumes of data, often including joins, aggregates, and groupings.
The goal of the SQL solution is to retrieve the number of page visits over specific time intervals, typically filtering or grouping by specific criteria like user ID, page URL, or visit timestamp. By efficiently using SQL Server's features like indexing and window functions, it's possible to achieve accurate and fast results.
Approach to SQL Query
Here is an overview of how to structure a typical solution for processing website traffic data:
- Data Filtering: Start by filtering out unnecessary data such as invalid records, incomplete sessions, or specific date ranges.
- Aggregation: Use SQL aggregate functions such as COUNT() and SUM() to summarize user visits based on the selected criteria.
- Join Tables: If you are working with multiple tables (e.g., traffic logs, user data), use appropriate JOINs to combine relevant information.
- Group Results: Group data by specific time intervals, such as hours or days, or by user characteristics such as geographical region.
Example SQL Query
Here's an example of a simple SQL query that counts website visits grouped by the day:
SELECT
CONVERT(date, VisitTimestamp) AS VisitDate,
COUNT(*) AS TotalVisits
FROM
WebsiteTraffic
GROUP BY
CONVERT(date, VisitTimestamp)
ORDER BY
VisitDate;
Important Points
It is crucial to properly index columns used in WHERE clauses or JOINs to improve query performance, especially when working with large traffic datasets.
The above query counts the total number of visits to the website for each day. By converting the timestamp into a date format, we group the data by day and order the results in ascending order. This approach can be extended to analyze user traffic by different time intervals, such as weekly or monthly reports.
Performance Considerations
For large-scale traffic data, SQL Server's performance can be optimized by:
- Using indexed views to pre-aggregate data for faster query execution.
- Implementing partitioned tables to store traffic logs by time intervals (e.g., monthly or yearly) for better management.
- Making use of SQL Server's window functions (e.g., ROW_NUMBER() or RANK()) to rank visits or analyze user behavior over time.
Conclusion
With a well-optimized SQL query and attention to performance, handling website traffic data in SQL Server can be both efficient and insightful. By leveraging the power of SQL Server's built-in functions, users can easily analyze traffic patterns, user behavior, and identify potential issues or opportunities for website improvement.
How to Approach SQL Challenges on Hackerrank Using SQL Server
Solving SQL challenges on platforms like Hackerrank requires a systematic approach, especially when working with SQL Server. The key to success is understanding how to implement SQL queries efficiently while considering the specific features and functions of SQL Server. The platform typically includes a set of predefined problems that you need to solve using various SQL concepts such as joins, aggregations, and subqueries.
To begin, make sure you familiarize yourself with the database schema and the problem requirements. SQL Server has specific syntax and functions that differ from other database management systems, so ensuring compatibility with SQL Server’s syntax is crucial. The Hackerrank editor will run your queries against a test database, so you need to write queries that are optimized and free from errors.
Steps to Solve SQL Challenges on Hackerrank
- Understand the Problem Statement: Carefully read the problem description and identify the tables, columns, and relationships that need to be queried.
- Familiarize Yourself with the Schema: Before writing any queries, understand the schema provided in the problem. This will help you avoid errors later on.
- Write and Test Queries: Start writing your queries. Ensure that they are optimized for performance, especially when working with large datasets. Use SQL Server-specific features such as window functions, CTEs, and common aggregation functions.
- Validate with Test Cases: Hackerrank will run multiple test cases to validate your solution. Always test your queries locally before submitting.
SQL Server provides several built-in functions and operations that can be helpful in solving specific tasks. Below are some of the commonly used SQL Server functions:
Function | Use Case |
---|---|
ROW_NUMBER() | Assigns a unique sequential integer to rows within a partition of a result set. |
CASE | Allows conditional logic within a query, similar to an if-else statement. |
DENSE_RANK() | Ranks rows without gaps in ranking values. |
Tip: When using SQL Server, be mindful of the differences between various SQL functions like COALESCE, ISNULL, and NULLIF to handle null values in your queries.
Optimizing SQL Server Queries for Faster Performance on Hackerrank
When working with SQL Server on platforms like Hackerrank, query optimization is crucial to ensure that solutions are both correct and efficient. Poorly optimized queries can lead to slow execution times, especially when dealing with large datasets. It's important to adopt strategies that reduce the overall query execution time and minimize resource consumption.
In this guide, we'll focus on a few critical techniques for improving SQL query performance on Hackerrank. These practices will help you solve challenges more effectively and improve your chances of passing time-limited tests.
Key Strategies for Query Optimization
- Use of Indexes: Indexes can significantly improve query performance by allowing faster data retrieval. Always ensure that the columns you filter or join on are indexed where possible.
- Limit the Result Set: Always use LIMIT or TOP clauses to restrict the number of rows returned, especially when testing on large datasets.
- Efficient Joins: Use inner joins over outer joins when possible, and ensure that join conditions are well-defined to avoid unnecessary data scanning.
- Proper Use of Aggregates: Avoid using COUNT(), SUM(), or other aggregation functions on large datasets unless absolutely necessary. Pre-filter data before applying aggregates to reduce the processing load.
Examples and Best Practices
- Using a simple WHERE clause to filter data early on in the query is an efficient practice.
- Using JOIN only when necessary, and ensuring that the join conditions are using indexed columns to minimize the scanning of unnecessary rows.
- Using GROUP BY after filtering and sorting your data, rather than before.
Optimizing SQL queries is an iterative process. Always test your queries with different data sizes and adjust indexes or logic accordingly.
Example of Optimized Query
Before Optimization | After Optimization |
---|---|
SELECT * FROM Orders WHERE OrderDate > '2020-01-01' |
SELECT OrderID, CustomerID FROM Orders WHERE OrderDate > '2020-01-01' |
Common Pitfalls When Writing SQL Queries for Coding Challenges
When tackling SQL challenges on platforms like HackerRank, developers often face several common issues that can hinder the efficiency and correctness of their queries. These pitfalls are sometimes subtle but can lead to incorrect results or inefficient code. Understanding these problems can save time and improve the overall approach to solving SQL challenges.
SQL queries for coding challenges need to be optimized, error-free, and well-structured. Mistakes in logic, syntax, or optimization can cause queries to fail or produce wrong results. Below are some of the most common errors developers make when writing SQL solutions.
1. Incorrect Use of Joins
- Misunderstanding the differences between INNER JOIN, LEFT JOIN, RIGHT JOIN, and FULL JOIN can lead to missing or incorrect data in the final result set.
- Using unnecessary joins can increase the time complexity, especially if the tables involved are large.
- Forgetting to apply proper filtering conditions in the WHERE clause, leading to data duplication or excessive rows in the output.
Tip: Always check if a join is necessary and if you're using the right type of join for the task. INNER JOIN often performs better than LEFT JOIN if you don't need to include unmatched rows from the left table.
2. Failure to Account for NULL Values
- Many challenges require handling NULL values explicitly. Not considering NULLs in the WHERE clause or in aggregate functions like COUNT() and SUM() can lead to incorrect results.
- Using IS NULL and IS NOT NULL conditions incorrectly can result in missing data or errors in data processing.
Important: Always account for NULL values when filtering or aggregating. Use COALESCE() or IFNULL() to handle NULLs effectively in queries.
3. Inefficient Query Structure
- Using SELECT * in large tables or complex queries can be inefficient, as it retrieves unnecessary columns and slows down the query execution.
- Not using indexes effectively in JOIN or WHERE conditions can lead to slow performance, especially with larger datasets.
- Complex subqueries that are not optimized can slow down the query and make the solution difficult to understand.
Common Inefficiencies | Best Practices |
---|---|
SELECT * | Specify only the columns you need to reduce data load and improve performance. |
Large subqueries | Consider using joins or breaking down subqueries into more manageable steps. |
Missing indexes | Ensure indexes are created on columns that are frequently queried or used in joins. |
Understanding the Most Common Data Types in SQL Server for Hackerrank
When working with SQL Server in the context of platforms like Hackerrank, understanding the various data types available is essential for writing efficient and accurate queries. SQL Server offers a variety of data types to store different kinds of data, and knowing which one to use can make your queries faster and more effective. It’s important to choose the appropriate data type depending on the data you are dealing with, as this can significantly impact performance, especially in large datasets.
In Hackerrank challenges, you will encounter different scenarios requiring the use of specific data types. Familiarity with these types not only helps you to solve problems quickly but also ensures you avoid errors that may arise from mismatched or incompatible data types. Below, we cover some of the most commonly used data types in SQL Server that you’ll likely encounter in these challenges.
Key Data Types to Know in SQL Server
- INT - Used to store integers, commonly used for primary keys or counting purposes.
- VARCHAR - A variable-length string type used for textual data where the length is unknown or variable.
- DATETIME - Used to store date and time values, including both date and time to a high degree of precision.
- DECIMAL - Used for precise numeric values, such as currency or exact calculations where floating-point numbers might introduce rounding errors.
It’s important to note that each of these types has specific storage requirements, and using them correctly will help you optimize your queries. For example, choosing INT for large numerical values when BIGINT would be more appropriate could lead to incorrect results or wasted space.
Always choose the data type based on the exact nature of the data. Using the wrong type can lead to data truncation, errors, or inefficiency in your queries.
Comparison of Common Data Types
Data Type | Storage Size | Usage |
---|---|---|
INT | 4 bytes | Stores integer values ranging from -2,147,483,648 to 2,147,483,647. |
VARCHAR | Variable | Stores variable-length strings, with a maximum length up to 8,000 characters. |
DATETIME | 8 bytes | Stores both date and time, accurate to 3 milliseconds. |
DECIMAL | Varies | Used to store numbers with exact precision, ideal for calculations like monetary values. |
How to Use SQL Joins Effectively in Hackerrank SQL Challenges
SQL joins are fundamental when solving problems in online coding platforms like HackerRank. They allow you to combine data from multiple tables based on shared attributes. When tackling SQL challenges, understanding how to use different types of joins can help you efficiently extract the needed information. Whether it’s an INNER JOIN, LEFT JOIN, or FULL JOIN, each type has specific use cases that can optimize your query performance.
Mastering joins in SQL not only enables you to solve more complex challenges but also improves the readability and efficiency of your code. In HackerRank SQL challenges, it’s essential to carefully select the right join type based on the problem requirements. Below, we explore some tips and strategies for effectively using SQL joins in your solutions.
Types of SQL Joins and Their Usage
Here’s a quick breakdown of the main types of joins and how they can be applied in SQL challenges:
- INNER JOIN: Retrieves records that have matching values in both tables. Ideal when you want to filter data based on common attributes.
- LEFT JOIN: Returns all records from the left table and matching records from the right table. If no match is found, NULL values are returned for the right table.
- RIGHT JOIN: Similar to the LEFT JOIN but returns all records from the right table and matching records from the left table.
- FULL JOIN: Combines results from both LEFT and RIGHT joins, returning all records when there is a match in either table.
Best Practices for Writing SQL Joins
- Identify the Problem Requirements: Carefully analyze the problem statement to determine which columns you need to combine and whether any filtering or aggregation is required.
- Use Aliases: When dealing with multiple tables, always use aliases to make your query more readable.
- Minimize Data Processing: Avoid using unnecessary joins. Only join tables that are necessary for the result you want.
- Optimize Performance: When dealing with large datasets, consider using specific join conditions to speed up your query.
Remember, SQL joins are most effective when used strategically, keeping performance in mind while ensuring correct results based on the problem's needs.
Example of SQL Join in a HackerRank Challenge
Here is an example of how you can use an SQL JOIN in a typical HackerRank challenge:
Table Name | Columns |
---|---|
Employees | emp_id, emp_name, department_id |
Departments | department_id, department_name |
In this scenario, you would use an INNER JOIN to match employees with their respective departments:
SELECT emp_name, department_name FROM Employees e INNER JOIN Departments d ON e.department_id = d.department_id;
Testing Your SQL Queries Locally Before Submission
When preparing SQL queries for platforms like Hackerrank, testing them locally is crucial to ensure they function correctly. Running queries in a local environment helps to spot potential issues early, such as syntax errors or incorrect logic. Testing your queries before submission not only saves time but also enhances the accuracy of your solution.
SQL Server offers various tools and environments that allow developers to test their queries effectively. By setting up a local instance of SQL Server, you can simulate the Hackerrank environment and troubleshoot your code efficiently. Below are some key steps to consider when testing your queries locally.
Steps for Testing SQL Queries
- Set Up SQL Server Locally: Install SQL Server on your machine or use SQL Server Express Edition for lightweight testing.
- Create the Same Database Schema: Replicate the database schema provided by Hackerrank to ensure compatibility with their test cases.
- Test Your Query: Run your query using sample data that mirrors the Hackerrank inputs as closely as possible.
- Check for Performance: Ensure your query performs optimally, especially with larger datasets.
- Fix Errors and Re-test: Identify and correct any errors in logic or syntax, then test again.
Common Issues When Testing Locally
- Data Mismatch: The test data on Hackerrank might differ from your local sample data, leading to unexpected results.
- SQL Compatibility: Some SQL functions or syntax may behave differently depending on the version of SQL Server used locally.
- Performance Issues: Query execution times might vary significantly depending on local hardware versus the Hackerrank server environment.
Always test your query with a variety of data sets, and keep in mind that even small discrepancies in data format or types can lead to issues in your final solution.
Helpful Tools for Testing
Tool | Purpose |
---|---|
SQL Server Management Studio (SSMS) | Full-featured environment for running and testing queries. |
SQL Server Profiler | Helps to identify performance bottlenecks and optimize queries. |
Visual Studio | Allows integration with SQL Server for debugging and testing queries in a development environment. |
Improving Query Readability and Debugging Tips for Hackerrank SQL Solutions
When working on SQL problems in platforms like Hackerrank, clarity and ease of understanding are crucial for both writing and debugging queries. While crafting a query, consider breaking it down into manageable parts, which makes it easier to trace the logic and identify any errors. This can help you spot issues early and improve your debugging process. In this context, simplifying complex expressions and using aliases meaningfully can significantly enhance query readability.
Moreover, debugging SQL queries requires systematic approaches to identify where things go wrong. This includes understanding the table structures, checking the logical flow of conditions, and validating results step by step. Here are some practical tips for improving both the readability of your SQL queries and the process of debugging them effectively:
1. Break Complex Queries into Smaller Pieces
Instead of writing one long and complex query, break it down into smaller, more manageable subqueries or Common Table Expressions (CTEs). This approach helps isolate potential issues and makes each part of the query easier to test individually.
- Use CTEs to break complex joins or filters into simpler chunks.
- Keep each subquery focused on a single task to enhance both clarity and reusability.
- Instead of nesting multiple layers of queries, split them logically for easier debugging.
2. Use Meaningful Aliases
When working with multiple tables in a query, always assign meaningful aliases to improve readability. Avoid using ambiguous or single-letter aliases that can confuse others (and yourself) during debugging.
Tip: Use descriptive aliases, such as
customer AS c
ororder_details AS od
, instead of generic aliases likea
orb
.
3. Debugging Techniques
Debugging in SQL often involves checking intermediate results and validating assumptions about data relationships. Below are some tips to debug SQL queries effectively:
- Start with simpler queries to ensure the base data is returned correctly before adding joins or complex conditions.
- Use
LIMIT
orTOP
to return only a small set of results, making it easier to spot issues. - Check the
EXPLAIN
plan to analyze the execution strategy of the query and identify inefficiencies.
4. Validate Using Sample Data
Use a small, controlled set of sample data to validate whether your query logic is functioning as expected. Testing with limited rows can help quickly identify logical flaws without running the risk of large-scale errors.
Table | Column | Sample Data |
---|---|---|
customers | id | 1, 2, 3 |
orders | customer_id | 1, 2 |
Handling Large Datasets and Complex SQL Queries on Hackerrank
Working with large datasets and intricate SQL queries can be challenging, especially when the data is massive or the query is highly complex. On platforms like Hackerrank, you must be strategic in optimizing your queries to ensure they run efficiently. This involves understanding indexing, query structuring, and optimizing join operations to reduce processing time.
Effective handling of these challenges requires a deep understanding of SQL functions, the right use of aggregate functions, and techniques such as subqueries and window functions. Here are some key strategies to approach these issues:
1. Efficient Query Structuring
When working with large datasets, it's crucial to structure your SQL queries in a way that minimizes resource consumption. Here are a few methods:
- Limit the dataset by using WHERE clauses early to filter out unnecessary data.
- Use proper indexes to speed up data retrieval, especially for large tables.
- Avoid SELECT *, and only retrieve the columns you actually need.
2. Optimizing Joins and Aggregations
Complex SQL queries often involve multiple joins and aggregations. Optimizing these operations can dramatically improve performance.
- Use INNER JOINs instead of OUTER JOINs when possible to reduce unnecessary data processing.
- Limit the rows being joined by filtering records first before performing joins.
- Leverage window functions (e.g., ROW_NUMBER(), RANK()) to handle complex aggregation operations more efficiently.
3. Working with Subqueries
Subqueries can be used to break down complex logic, but be mindful of their impact on performance.
Subquery Type | Performance Tip |
---|---|
Correlated Subqueries | Can be slow due to multiple executions. Try to replace them with joins or common table expressions (CTEs). |
Non-Correlated Subqueries | Usually faster; ensure that the subquery is optimized with indexes. |
Always check query execution plans and experiment with different strategies to identify the most efficient approach when dealing with large datasets.