Vuejs Push Notification
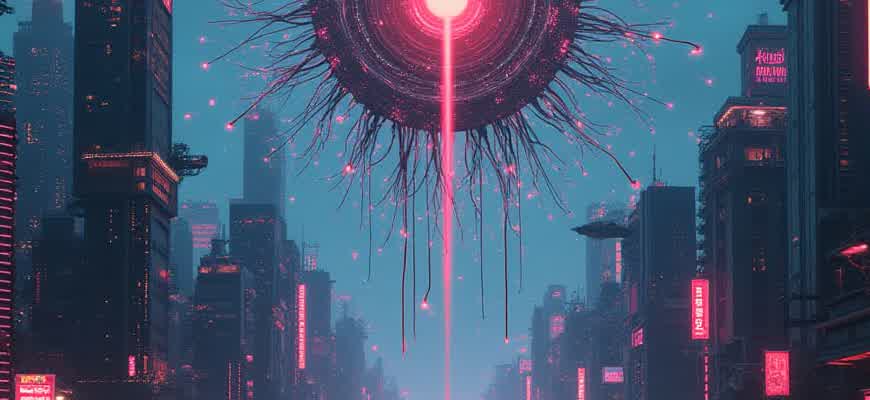
Push notifications are an essential feature for keeping users engaged with web applications. In Vue.js, setting up push notifications involves several key steps to ensure seamless communication between the client and the server. Here's a breakdown of the process:
- Configure the service worker to handle push notifications.
- Use the Push API to request permission from the user.
- Set up a backend to send the push messages to users' devices.
Important: The Push API relies on the Service Worker API, which must be supported by the user's browser.
The process begins with registering a service worker in your Vue.js app. This worker acts as an intermediary, handling push events in the background. Once the service worker is set up, the next step is to request the user's permission for receiving notifications. After obtaining consent, the server can push notifications to the client, which will be displayed based on the configuration set in the service worker.
Steps for Vue.js Push Notification Setup
Step | Description |
---|---|
1. Register Service Worker | Implement the service worker script in your app to handle push events. |
2. Request User Permission | Prompt the user for permission to display notifications. |
3. Configure Backend | Set up a server to send push notifications to subscribed clients. |
Vue.js Push Notification: A Practical Guide for Implementation and Optimization
Integrating push notifications into a Vue.js application can significantly enhance user engagement. These notifications can be used for a variety of purposes, including real-time updates, reminders, and personalized alerts. The process involves configuring both the front-end and back-end components, ensuring smooth interaction with service workers and handling notifications efficiently.
In this guide, we will walk through the implementation process, from setting up the necessary dependencies to optimizing notification delivery. By the end of this tutorial, you'll be able to integrate notifications into your Vue.js application seamlessly and apply performance optimizations for better user experience.
Steps for Implementation
- Setting up the Service Worker
- Configuring Push Notifications API
- Handling Permissions and User Consent
- Displaying Notifications in Vue.js
- Optimizing Notification Payloads
The integration of push notifications begins with configuring the service worker, which acts as the intermediary between your Vue.js app and the push service. This allows you to receive notifications even when the application is not open in the browser.
Important: Make sure that the service worker is registered before requesting permission to send notifications. This ensures that the push subscription can be successfully created.
Optimizing Push Notifications
To avoid overloading users with irrelevant messages, optimize push notification payloads. This includes:
- Minimizing the size of notification content
- Segmenting your audience to send targeted messages
- Scheduling notifications at appropriate times to increase user engagement
Additionally, consider user preferences for notification types and frequency. Providing users with control over the kinds of notifications they receive can increase retention and reduce opt-out rates.
Optimization Factor | Best Practice |
---|---|
Payload Size | Keep the notification content concise (under 4KB) |
Targeting | Segment users based on behavior or demographics |
Frequency | Send notifications at times of day with higher engagement |
How to Add Push Notifications to Your Vue.js Project
Integrating push notifications into a Vue.js application enhances user engagement and allows for real-time communication. These notifications can be implemented using the browser’s Notification API combined with a service worker for background handling. This guide will walk you through the process step by step.
Before you start, make sure your app is served over HTTPS, as service workers require a secure context. Additionally, you’ll need a push notification service, such as Firebase Cloud Messaging (FCM), to handle push messages.
Steps to Implement Push Notifications
- Set up the Push Notification Service: Register for a push notification service like FCM. Obtain the necessary keys (e.g., VAPID keys for web push). This will allow your app to send push notifications.
- Register the Service Worker: In your Vue.js app, create a service worker file. This file will handle the reception of push messages and display notifications to users even when they are not actively using the app.
- Request Permission: Ask users for permission to send them push notifications. This is done using the Notification API.
- Subscribe to Push Notifications: After getting permission, use the PushManager API to subscribe the user to push notifications. Store the subscription object on the server for later use when sending notifications.
Important: Always inform users about the nature of notifications and provide them an option to opt-out at any time.
Service Worker Example
navigator.serviceWorker.register('/service-worker.js') .then(function(registration) { console.log('Service Worker registered with scope:', registration.scope); }) .catch(function(error) { console.log('Service Worker registration failed:', error); });
Table: Service Worker Push Event
Event | Description |
---|---|
push | Triggered when a push message is received. |
notificationclick | Fires when a user clicks on a notification. |
Configuring Service Worker for Push Notifications in Vue.js
Integrating push notifications in a Vue.js application requires setting up a service worker that will manage background tasks like receiving notifications. The service worker is a script that runs in the background, separate from the web page, and can respond to events such as push notifications. In this process, the first step is registering the service worker in the Vue.js app.
The service worker must be configured properly to handle push notifications. This involves several steps, including managing subscription information and handling events related to push messages. Below is a guide on how to configure the service worker for Vue.js push notifications.
Steps to Configure the Service Worker
- Register the Service Worker: The first step is to register the service worker in your Vue.js app. This is typically done in the main.js or App.vue file.
- Request Permission for Notifications: Before pushing notifications, you need to ask the user for permission to send notifications. Use the Notification.requestPermission() method to do this.
- Subscribe to Push Service: After getting permission, subscribe the user to a push service. You will need to generate a push subscription object, which can be done through the serviceWorker.pushManager.subscribe() method.
Service Worker Code Example
Below is an example of how to configure the service worker to handle push notifications:
self.addEventListener('push', function(event) {
const options = {
body: event.data.text(),
icon: 'images/icon.png',
badge: 'images/badge.png'
};
event.waitUntil(
self.registration.showNotification('Push Notification', options)
);
});
Important Information
Make sure that the service worker is registered in the root directory of your application to ensure it can handle push events properly.
Table: Key Service Worker Methods
Method | Description |
---|---|
self.addEventListener('push') | Handles incoming push notifications |
self.registration.showNotification() | Displays a notification to the user |
self.registration.pushManager.subscribe() | Subscribes the user to a push service |
Setting Push Notification Permissions in Vue.js for Various Browsers
In order to enable push notifications in a Vue.js application, it's crucial to properly set up permissions across different browsers. Push notifications rely on the browser's permission system, which varies between environments. Some browsers, such as Chrome and Firefox, handle permission requests similarly, while others like Safari require additional setup due to their unique implementation of the push API.
To ensure smooth functionality, developers need to account for these variations and handle permissions in a way that is compatible with most users. The process typically involves detecting the user's browser, checking for notification support, and requesting permission when needed. Below, we’ll go through the key considerations for setting permissions for various browsers and how to adapt the implementation in Vue.js.
1. Browser-Specific Permission Requests
Different browsers handle the notification permission flow in different ways. Here's a summary of how you can handle permission requests for the most popular browsers:
- Chrome/Firefox: Both browsers support the Push API with relatively similar permission prompts. The browser will ask the user whether they want to allow or deny notifications.
- Safari: Safari requires users to opt-in to push notifications through the Apple Push Notification Service (APNS). The notification permission request must be triggered by a user interaction (like a click), and Apple uses a more restrictive approach in handling permissions.
- Edge: Microsoft Edge follows a similar flow to Chrome, allowing for push notification permissions and supporting the Push API for web apps.
2. Implementing Permission Request in Vue.js
In Vue.js, managing permissions is straightforward but needs some conditional checks to account for browser differences. Here's an example of how to request notification permissions:
if ("Notification" in window) {
if (Notification.permission === "default") {
Notification.requestPermission().then(permission => {
if (permission === "granted") {
console.log("Push notification permission granted!");
} else {
console.log("Push notification permission denied.");
}
});
}
}
3. Key Browser Features
When implementing push notifications, you may encounter differences between browsers in terms of support and behavior. Here’s a quick reference:
Browser | Supports Push Notifications | Permission Flow |
---|---|---|
Chrome | Yes | User is prompted to allow or deny |
Firefox | Yes | User is prompted to allow or deny |
Safari | Yes, with APNS | Requires user interaction for permission |
Edge | Yes | User is prompted to allow or deny |
Important: Always check if the browser supports notifications before requesting permission. This ensures a smooth user experience and avoids errors.
Customizing Push Notification Appearance in Vue.js
In Vue.js, customizing the look of push notifications involves manipulating the visual components using various techniques, ranging from adding custom icons to adjusting the layout and colors. This ensures notifications align with your application's design, providing a seamless user experience. By utilizing the Push API and service workers, you can not only control notification content but also modify their appearance and behavior to match your app's aesthetic.
Vue allows developers to customize notifications in a way that they appear cohesive with the overall UI of the application. The integration of push notifications can be enhanced by incorporating specific styles and actions like buttons, links, and actions that users can interact with directly from the notification.
Key Customization Techniques
- Custom Icons: Use your app's branding elements to create a unique notification icon.
- Notification Body: Control the message's font size, color, and style to suit the design guidelines.
- Buttons: Include action buttons with custom labels to enhance interactivity.
Steps for Styling Notifications
- Define the visual properties in the service worker when setting up notifications.
- Use the Notification API's options to adjust title, body, icon, and actions.
- Modify appearance via CSS to ensure notifications reflect the app’s theme.
Note: Service workers can be used to define the appearance of notifications, but additional styling should be done using JavaScript and CSS to ensure consistency across different devices and browsers.
Example Configuration
Property | Description | Example Value |
---|---|---|
title | Defines the notification’s title. | "New message" |
body | The main content of the notification. | "You have a new message from John" |
icon | Specifies the image displayed as the notification icon. | "images/icon.png" |
actions | Defines buttons or actions to be taken. | [{action: "view", title: "View", icon: "images/view.png"}] |
Managing Push Notification Data and Payloads in Vue.js
When integrating push notifications in Vue.js applications, handling the incoming data and payloads is crucial for providing an optimal user experience. Push notifications typically contain a payload with various data, such as titles, bodies, and additional metadata. To effectively process and display this information, developers need to extract and manipulate the payload before using it in the Vue.js application.
The payload structure can vary depending on the service, but the general approach to handling it remains similar. Typically, push notifications contain two parts: the notification object (which includes the title, message, and other display elements) and the data object (which may contain extra information relevant to the application logic). Let's explore how to properly manage and display this data within a Vue.js environment.
Understanding Push Notification Payload Structure
Push notifications typically follow a standard format, with key-value pairs that store essential information. Below is an example of a typical notification payload:
Key | Value |
---|---|
title | The title of the notification |
body | The message content of the notification |
data | Additional metadata or custom data for the app |
Extracting and Handling Notification Data in Vue.js
In a Vue.js application, push notifications can be processed through the Service Worker. Upon receiving a push message, the service worker will handle the payload and pass it to the main application. Here’s how you can access and display the notification data:
- Service Worker Registration: Ensure that the service worker is properly registered and listens for push events.
- Push Event Listener: Inside the service worker, implement a listener for the push event that will handle the incoming notification.
- Display Notification: Use the data from the payload to customize the notification display to the user.
Important: Always validate the payload structure before processing it in the Vue component to prevent errors and ensure the data is correctly handled.
Custom Data and Actions
Often, push notifications include custom data that the application can use to trigger specific actions or navigate to a particular view. This is typically included in the data object of the payload. For example:
- Display a modal with additional content.
- Redirect the user to a specific route in the app.
- Update the app’s state or trigger certain background tasks.
By using this data, Vue.js components can react dynamically to the received notifications, providing a more interactive and responsive user experience.
Debugging Push Notifications in Vue.js: Common Issues and Fixes
When working with push notifications in Vue.js, developers may encounter several challenges that can prevent notifications from working properly. Understanding the most common issues and knowing how to resolve them is key to building a reliable notification system. This article will cover some of the most frequent problems developers face, along with solutions to debug and fix them effectively.
Push notifications are a critical feature in modern web applications, but they rely on multiple components such as service workers, browser compatibility, and correct API calls. Below are some typical problems and the steps you can take to solve them.
1. Service Worker Issues
Service workers are essential for handling push notifications in Vue.js. If your service worker isn't registered or activated properly, notifications won't be received. Here's how to check:
- Ensure the service worker file is in the correct directory (typically in the root of your project).
- Check if the service worker is being registered using the correct path.
- Verify that the service worker is not being blocked by the browser.
Important: To ensure the service worker is active, use browser developer tools to check the "Service Workers" tab for any issues.
2. Incorrect Push Notification Permissions
If the user hasn't granted permission for push notifications, your application won't be able to display them. You can verify and manage permission states with the following steps:
- Check if permission is granted by calling
Notification.permission
. - If the permission is "default" or "denied," prompt the user to allow notifications.
- Handle the cases when permission is not granted and notify the user accordingly.
3. Issues with API Payload
The payload of the push notification might not be correctly formatted, causing the notification to fail. Make sure the following points are covered:
Check | Solution |
---|---|
Payload Structure | Ensure the notification payload contains necessary fields like title, body, and icon. |
Encoding | Ensure proper encoding of the payload to avoid corrupted characters. |
By following these steps, you'll be able to debug common issues related to push notifications in Vue.js applications, ensuring a smooth and reliable user experience.
Optimizing Push Notification Delivery in Vue.js for High Engagement
Push notifications can significantly enhance user interaction when implemented properly in a Vue.js application. However, to maintain high engagement, the delivery of these notifications needs to be fine-tuned. Achieving optimal notification delivery involves understanding both the technical setup and user behavior to deliver messages that are timely, relevant, and non-intrusive.
By focusing on message relevance, timing, and personalized content, developers can ensure that notifications are not only delivered efficiently but also engage users effectively, resulting in higher response rates.
Strategies for Optimized Notification Delivery
Several strategies can be employed to optimize the delivery of push notifications in Vue.js applications:
- Tailored Content: Use data-driven insights to personalize notifications, ensuring they meet individual user needs and preferences.
- Optimal Timing: Schedule notifications based on user activity patterns to increase the likelihood of interactions.
- Effective Segmentation: Group users based on demographics or behavior to send more targeted and relevant messages.
Personalized notifications that align with user preferences have a far greater chance of increasing engagement than generic messages.
Improving Delivery with Technical Approaches
To further improve notification delivery, it is essential to leverage reliable technical solutions:
- Service Workers: Implement service workers for handling background notifications, ensuring messages are delivered even when the app is not active.
- Network Resilience: Set up retry mechanisms to ensure messages are sent even if there are temporary issues with the network or connection.
- Leverage Firebase Cloud Messaging: Utilize Firebase to manage the push notification infrastructure, providing scalable and efficient message delivery.
Analyzing Notification Effectiveness
Tracking the performance of notifications is crucial to refining delivery strategies. The following metrics can help measure the success of push notifications:
Metric | Purpose | Tools |
---|---|---|
Delivery Rate | Tracks how many notifications are successfully delivered to users. | Firebase, Google Analytics |
Engagement Rate | Measures user interaction with notifications. | Firebase Analytics, Mixpanel |
Conversion Rate | Measures the number of users taking desired actions after interacting with notifications. | Google Analytics, Firebase Analytics |
Consistent monitoring of notification performance helps fine-tune strategies, resulting in better user engagement and overall application success.
Scaling Push Notification Infrastructure for Vue.js Applications
When building Vue.js applications, ensuring scalable push notification infrastructure is essential to maintain performance and reliability. As user bases grow and the number of notifications increases, it's critical to design an architecture that can handle the load effectively without compromising the user experience. This involves carefully considering message delivery mechanisms, backend services, and resource optimization.
To achieve scalability, developers need to focus on using efficient messaging protocols and distributing workloads across multiple servers or services. The use of cloud services or dedicated notification services can alleviate some of the strain, ensuring that the system can scale seamlessly as demand rises.
Key Strategies for Scaling
- Optimize Message Queues: Utilize message queuing systems like Kafka or RabbitMQ to buffer messages and process them asynchronously. This prevents server overload and ensures reliable delivery even during peak usage times.
- Leverage Cloud-Based Solutions: Cloud services such as Firebase or AWS SNS (Simple Notification Service) provide scalable infrastructure for push notifications, with built-in redundancy and load balancing.
- Implement Rate Limiting: To prevent notification spamming and manage server resources, implement rate limiting for both push notifications and background tasks.
- Distributed Push Servers: Distribute push notification processing across multiple servers or services to avoid single points of failure and increase fault tolerance.
By distributing the workload across multiple regions and implementing automatic scaling, push notification systems can efficiently handle large volumes of users and messages without performance degradation.
Infrastructure Components for Push Notifications
Component | Description |
---|---|
Notification Service | Handles the core functionality of pushing messages to users, such as Firebase Cloud Messaging (FCM) or AWS SNS. |
Message Queue | Buffers push notification requests and allows for asynchronous processing, reducing server load. |
Backend APIs | Handles the logic for managing user subscriptions, push triggers, and delivering notifications to the appropriate service. |
Analytics Service | Tracks push notification delivery and engagement metrics, allowing developers to optimize content and frequency. |