Javascript Push Notifications
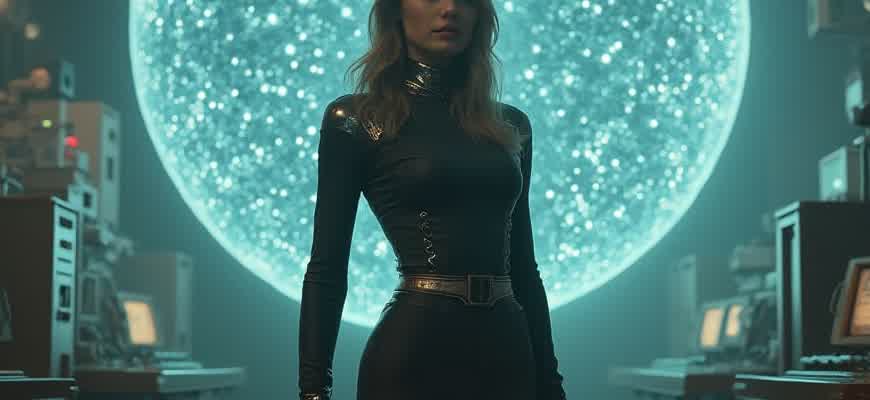
Push notifications have become a key element of modern web applications, allowing websites to send real-time updates to users even when they are not actively interacting with the page. In this article, we explore how JavaScript can be used to implement and manage push notifications, making it easier for developers to engage users with relevant content and alerts.
Overview of Push Notification Process
- User subscribes to notifications via the browser.
- The web server sends notifications to the user through the push service.
- The user's browser displays the notification even if the site is not open.
Push notifications offer a powerful way to re-engage users and increase retention by delivering timely and relevant updates directly to their devices.
Key Components in Push Notification Flow
- Service Worker: Handles background tasks and manages push events.
- Push Manager: Allows the subscription process and notification delivery.
- Push API: Provides the functionality to send push messages.
Example of Push Subscription Process
Step | Description |
---|---|
1. User Permission | The user is asked to allow notifications. |
2. Subscription | The client subscribes to push notifications through the Push API. |
3. Notification Delivery | The server sends notifications, and the service worker displays them. |
Boosting User Engagement with JavaScript Push Notifications
Push notifications are a powerful tool for driving user engagement on web applications. By sending timely updates directly to users' devices, you can increase retention, prompt action, and enhance the overall user experience. JavaScript makes it easy to implement these notifications, leveraging the capabilities of the Notification API and Service Workers.
To effectively use push notifications, it's important to focus on personalization and timing. Custom messages tailored to user preferences can make a significant impact, while ensuring notifications are sent at optimal times increases the likelihood of user interaction.
Steps to Implement JavaScript Push Notifications
- Step 1: Request permission from the user to send notifications. This is done through the Notification API.
- Step 2: Set up a service worker to handle background tasks like sending and receiving push messages.
- Step 3: Use the Push API to send push notifications to users, either manually or through a server-side system.
Key Considerations:
Personalized content and timely delivery are essential for effective push notifications. Generic messages tend to be ignored, whereas customized alerts related to the user's interests or behavior lead to better engagement rates.
Best Practices for Maximizing User Engagement
- Segment your audience: Use data to identify user segments and tailor messages accordingly.
- Send relevant updates: Notify users about offers, reminders, or new features based on their activity or preferences.
- Don't overdo it: Too many notifications can lead to user fatigue and prompt them to unsubscribe.
Comparison of Notification Delivery Options
Method | Advantages | Challenges |
---|---|---|
Push Notifications | Direct engagement, real-time updates, customizable | Permission-based, can be intrusive if overused |
Email Notifications | Longer message formats, easier to implement | Delayed delivery, lower open rates |
In-app Notifications | Great for active users, integrates with app features | Limited to app usage, not as visible |
Integrating Push Notifications with Your Web Application
Push notifications allow web applications to send timely alerts directly to users, even when the application is not open. By integrating this feature, developers can keep users engaged and informed about updates, news, or promotions in real-time. This integration requires several steps, including enabling the necessary permissions, setting up a service worker, and configuring a push notification service. Here's a closer look at how to implement it effectively.
To begin the integration, you will need to handle both the frontend and backend parts. On the frontend, you must request permission from the user to show notifications. Once the user grants permission, the browser will register a service worker, which acts as a bridge for receiving notifications. On the backend, you will need to configure a push notification service, such as Firebase Cloud Messaging, to send messages to the user.
Steps to Integrate Push Notifications
- Request User Permission: Ask users for permission to send notifications, usually by using the Notification API.
- Register Service Worker: The service worker allows the browser to receive push messages even when the application is closed.
- Set Up Backend Service: Use services like Firebase or your custom server to send push messages to the user's device.
- Send Push Notifications: When the server triggers a push event, the service worker handles displaying the notification on the user's screen.
Keep in mind that each browser and platform has its own set of rules for push notifications, so testing on different devices is essential.
Code Example for Push Notification
Step | Code Snippet |
---|---|
Request Permission | Notification.requestPermission().then(permission => { console.log(permission); }); |
Register Service Worker | navigator.serviceWorker.register('/service-worker.js'); |
Send Push Notification | self.registration.showNotification('New Message', { body: 'You have a new message!' }); |
With the service worker set up and the backend ready, you can begin sending notifications to users. The process might require some debugging and careful handling of user permissions, but once completed, it can significantly enhance user engagement with your application.
Personalizing Push Notification Content Based on User Actions
To enhance user engagement, push notifications should be tailored to reflect individual behavior, making the messages more relevant and timely. By analyzing user activity and preferences, you can send targeted messages that increase interaction rates and improve the overall user experience. This customization ensures that notifications are not perceived as spam but as valuable, personalized communication.
Customizing push notifications allows for better user retention, as the content can be fine-tuned according to the actions users take within an app or website. For example, sending a special offer based on past purchases or reminding users about abandoned carts can significantly boost conversion rates. The following are some key strategies for creating personalized notifications.
Behavior-Based Notification Strategies
- Purchase History: Send targeted offers based on the products a user has previously purchased.
- Location Data: Use geographical location to offer relevant deals or events nearby.
- Activity Level: Engage inactive users with special messages or reminders.
- Time Sensitivity: Customize notifications to be sent at the most effective times, based on the user's behavior patterns.
Dynamic Notification Content
By integrating dynamic content into push notifications, developers can personalize the messaging even further. This could include displaying a user’s name, specific product recommendations, or updates on activities they’ve shown interest in.
Behavior | Notification Content | Goal |
---|---|---|
Recent Browsing | “You left something behind! Check out your recent browsing history.” | Encourage return visits and conversions. |
Location-Based | “A special offer is available at a store near you!” | Increase foot traffic and local engagement. |
Inactive User | “We miss you! Here’s 20% off your next purchase.” | Re-engage and bring users back into the app. |
Custom push notifications are not just about sending messages; they are about sending the right message at the right time to the right user.
Configuring Push Notification Permissions Across Browsers
For web push notifications to work, it’s essential to obtain permission from users through the browser. The process varies slightly between different browsers, but the core idea remains the same: you need to ask for permission to send notifications. Below is a guide that highlights how to handle these permissions for popular browsers.
Each browser has its own method of requesting and handling push notification permissions. While the general workflow is similar, there are specific nuances to consider, including differences in prompt UI and user interaction. Understanding these differences will help ensure a smooth experience for your users.
Permission Requests in Different Browsers
- Chrome: Chrome uses a simple permission request, which pops up when you attempt to subscribe the user to push notifications. If the user grants permission, your service worker will be activated to receive push notifications.
- Firefox: Firefox displays a notification permission prompt only when the user clicks on a button for subscription. The permissions can also be managed manually via browser settings.
- Safari: Safari uses a slightly different approach. It requires user interaction to trigger the notification permission prompt, and permissions are set per website.
Steps for Requesting Permission
- Check if Push Notifications are Supported: Before requesting permission, verify that the browser supports push notifications. For example, use
if ('PushManager' in window)
to check availability in the current browser. - Request Permission: Use the
Notification.requestPermission()
method to ask the user for permission to send notifications. Handle the return value to adjust the flow accordingly (granted, denied, or default). - Handle Responses: Depending on the user's decision, either proceed with subscribing to push notifications or handle the case where they deny the request.
Permissions Table
Browser | Permission Prompt Behavior | Manual Configuration |
---|---|---|
Chrome | Automatic prompt on page load | Settings > Privacy and Security > Site Settings > Notifications |
Firefox | Prompt after user interaction | Settings > Privacy & Security > Notifications |
Safari | Triggered by user interaction | Preferences > Websites > Notifications |
Important: Always consider providing clear and concise information about why you're requesting permission, as users are more likely to accept notifications if they understand the benefit.
Optimizing Push Notification Delivery for Mobile and Desktop Users
When dealing with push notifications, it is crucial to ensure that the messages reach users efficiently, regardless of the platform they are on. Whether targeting mobile or desktop users, optimizing delivery involves various technical approaches that cater to the distinct characteristics of each device. Below, we outline key strategies for enhancing push notification performance for both environments.
Different devices have specific constraints and opportunities. For mobile users, factors like battery life, data usage, and connectivity can impact the effectiveness of push notifications. In contrast, desktop users typically have fewer limitations but may be less engaged, requiring a more personalized approach to grab attention. This section explores strategies for fine-tuning push notification delivery across platforms.
Key Optimization Strategies
- Device-Specific Payloads: Tailor your notification content based on the device type. For mobile devices, minimize payload size to save on data usage and reduce battery consumption. Desktop notifications, on the other hand, can be more content-rich, given fewer limitations.
- Time Zone Adjustments: Consider the user’s location to deliver notifications at the optimal time. Push notifications sent during off-hours might be ignored, leading to poor engagement rates.
- Background Syncing: For mobile devices, ensure background syncing is efficient to avoid unnecessary delays in notification delivery. Use service workers to manage notifications in the background while keeping battery usage low.
Device-Specific Delivery Mechanisms
- Mobile Devices: Use the Push API with a Service Worker to manage notification delivery even when the app is not actively running.
- Desktop Devices: Implement browser-specific push protocols like Web Push for Chrome or Firefox to ensure smooth and consistent delivery.
- Fallback Strategies: On both platforms, ensure that there are fallback mechanisms for when a notification fails to deliver, such as retries or alternative channels.
Delivery Performance Metrics
Metric | Mobile | Desktop |
---|---|---|
Open Rate | Higher engagement is often seen on mobile, but notifications need to be concise. | Desktop users may ignore notifications unless they are highly relevant or time-sensitive. |
Delivery Speed | Mobile devices may experience delays due to network and battery limitations. | Desktop notifications typically have faster delivery times, as the device is often connected to a stable internet connection. |
Failure Rate | Higher failure rate on mobile due to connectivity and battery constraints. | Failure rates are lower, but push notifications may be blocked by browsers or operating systems. |
Important: Always test your push notifications on both mobile and desktop platforms before deploying them widely. Different devices may have subtle differences in performance that could affect user engagement.
Analyzing User Engagement with Push Notifications: Tracking Interactions and Conversions
Understanding how users engage with push notifications is crucial for improving their effectiveness. By tracking user interactions, such as clicks, views, and conversions, businesses can gain valuable insights into user behavior. This data helps refine notification strategies and ensures that content reaches its intended audience with optimal impact.
Monitoring push notification performance involves analyzing various metrics to determine the overall success of campaigns. Metrics like click-through rates (CTR), conversion rates, and user retention are key indicators that provide a clear picture of engagement and effectiveness.
Tracking User Clicks and Conversions
Tracking user interactions with notifications can provide insight into their effectiveness in driving actions. Key performance indicators (KPIs) include:
- Click-through Rate (CTR) – The percentage of users who click on the notification after receiving it.
- Conversion Rate – The percentage of users who take a desired action (e.g., purchase, sign-up) after interacting with the notification.
- Retention Rate – The percentage of users who continue to engage with the platform after receiving push notifications.
By tracking these metrics, businesses can identify patterns and adjust their notification strategies to improve user engagement.
Using Analytics to Optimize Notifications
Effective push notification campaigns rely on continuous analysis and optimization. Tools that track user behavior can provide detailed data for decision-making. Below is a table that outlines some key tools and features to consider:
Tool | Features |
---|---|
Google Analytics | Real-time tracking, conversion tracking, user behavior analysis |
Firebase | Push notification segmentation, A/B testing, campaign analytics |
OneSignal | Click tracking, conversion tracking, user engagement insights |
Tip: A/B testing notifications with different messaging or timing can significantly impact conversion rates and user engagement.
Ensuring Privacy and Security in JavaScript Push Notifications
Push notifications are a powerful tool for engaging users, but they can also pose significant privacy and security risks if not implemented carefully. Ensuring that users’ data is handled securely and that their privacy is respected is crucial for any web application utilizing push notifications. Several practices should be followed to ensure that notifications are sent safely and that users' information remains private.
To effectively secure push notifications, developers must address both the technical implementation and user consent. The security measures should include encrypting communication channels, validating users' identity, and limiting the amount of personal data shared through notifications.
Best Practices for Securing Push Notifications
- Use Secure Channels: All push notifications should be sent through HTTPS connections to ensure that data is transmitted securely. This prevents interception and tampering by third parties.
- Authenticate Users: Implement a reliable authentication system to verify that the recipient of the notification is the intended user. Techniques such as token-based authentication and OAuth can enhance security.
- Limit Data Exposure: Avoid sending sensitive personal information within the notification content. Instead, provide a link to a secure page where the user can access the information securely.
Considerations for User Privacy
When requesting permission to send push notifications, ensure users are fully informed about the types of notifications they will receive and the data that will be collected. Always respect user preferences and provide an easy way to opt-out.
- Inform Users Clearly: Always inform users about what kind of data will be collected and how it will be used before requesting permission for push notifications.
- Offer Opt-out Options: Ensure users can easily unsubscribe from notifications at any time without losing access to core functionalities of the website or application.
- Comply with Regulations: Adhere to privacy laws such as GDPR and CCPA to ensure that user data is handled responsibly and transparently.
Security Measures in Push Notification Systems
Security Aspect | Action |
---|---|
Data Encryption | Use HTTPS and encrypt payload data to prevent unauthorized access. |
Token Validation | Verify token authenticity before sending notifications. |
Data Minimization | Avoid sending unnecessary personal information in push messages. |
Automating Push Notification Campaigns with Scheduled Delivery
Push notifications offer an efficient way to engage users, but for businesses with large audiences, manually sending these notifications can be time-consuming. Scheduling the delivery of notifications allows marketers to automate campaigns, ensuring timely and relevant content reaches users without constant oversight. This method not only saves time but also improves the targeting of notifications based on user behavior and preferences.
By automating push notification campaigns, businesses can ensure that notifications are delivered at the most effective times. Scheduled delivery enables segmentation of audiences based on time zones, user activity, and other specific criteria. This approach maximizes user engagement while reducing the risk of sending irrelevant or poorly timed messages.
Advantages of Scheduled Push Notifications
- Time efficiency: Marketers can set up campaigns in advance, freeing up time for other tasks.
- Targeted delivery: Notifications can be sent at optimal times based on user behavior or preferences.
- Consistency: Scheduled notifications ensure that users receive timely updates even during off-hours.
Best Practices for Automated Campaigns
- Segment your audience: Customize messages based on specific user groups for better relevance.
- Analyze optimal delivery times: Review user activity patterns to determine the best time for sending notifications.
- Test and iterate: Continuously analyze the performance of your notifications and refine the scheduling strategy.
Scheduled delivery helps ensure push notifications are received when users are most likely to engage, improving the overall effectiveness of campaigns.
Example of a Notification Schedule
Time Zone | Segment | Notification Type |
---|---|---|
GMT +3 | Active Users | Product Update |
GMT +1 | New Subscribers | Welcome Message |
GMT +5 | Inactive Users | Re-engagement Offer |
Testing Push Notification Performance Across Multiple Devices
When testing push notifications in a JavaScript-based system, evaluating performance across a variety of devices is crucial. Different devices, operating systems, and browser configurations can lead to varying results. Performance testing ensures that your notifications are delivered on time, display properly, and do not negatively affect the user experience across multiple platforms.
Device diversity presents challenges in terms of push notification reliability and behavior. Therefore, testing should include not only the success rate of notification delivery but also the performance of notification display, including load times, interactivity, and battery consumption across devices such as smartphones, tablets, and desktops.
Key Aspects to Consider
- Device Variability: Notifications may behave differently on iOS vs. Android or across different browser versions.
- Network Conditions: Slow or intermittent connections can affect delivery speed and reliability.
- Battery Impact: Push notifications should not heavily drain battery life, particularly on mobile devices.
Testing Methodology
- Cross-Platform Testing: Ensure notifications are tested across multiple platforms like Android, iOS, and desktop (Chrome, Firefox, etc.).
- Load Testing: Simulate different network conditions and large volumes of notifications to see how the system handles scalability.
- Time-to-Delivery Monitoring: Track the time it takes for notifications to be delivered and displayed, comparing results on various devices.
Performance Test Example
Device | Delivery Time (Seconds) | Battery Impact (%) | Notification Display Time (Seconds) |
---|---|---|---|
iPhone 12 (iOS) | 3 | 5 | 2 |
Samsung Galaxy S21 (Android) | 2 | 4 | 1.5 |
Desktop (Chrome) | 1 | 1 | 0.5 |
Remember, performance can differ significantly depending on user settings, so it's essential to perform testing on real devices rather than relying solely on simulators or emulators.