Web Performance Optimization Techniques
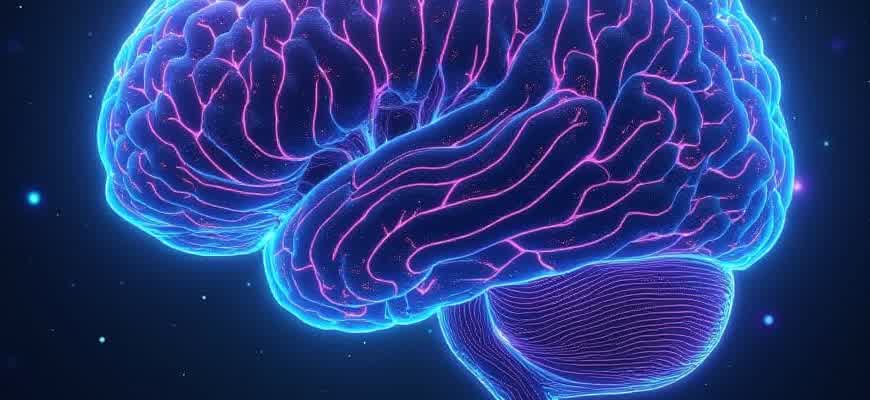
Improving website speed is essential for delivering a seamless user experience. Several methods can be employed to reduce page load times and enhance overall performance. These strategies focus on optimizing the assets, reducing server response time, and improving client-side rendering efficiency.
Key takeaway: Faster websites not only improve user engagement but also contribute to better search engine rankings.
Optimization Techniques
- Minifying resources: Reducing the size of JavaScript, CSS, and HTML files by removing unnecessary spaces, comments, and unused code.
- Image optimization: Compressing images without losing quality to decrease load times.
- Lazy loading: Deferring the loading of images and other media files until they are needed, thus speeding up the initial page load.
Server-Side Enhancements
- Server-side caching: Storing static resources or database queries on the server to reduce processing time for frequently requested data.
- Content Delivery Networks (CDNs): Distributing content across multiple geographically located servers to ensure faster access for users from different regions.
Table of Key Optimization Factors
Technique | Impact |
---|---|
Minification | Reduces file size, improving download time. |
Lazy Loading | Improves initial load by deferring non-critical resources. |
CDN Usage | Speeds up content delivery across different regions. |
Strategies to Reduce JavaScript Execution Time for Quicker Page Loads
Minimizing JavaScript execution time is crucial for improving page load performance. By optimizing how and when scripts are executed, web developers can ensure faster rendering, reduced latency, and a smoother user experience. One of the primary causes of slow page loads is excessive or inefficient JavaScript processing, which can block other critical operations like rendering and network requests. Fortunately, there are several techniques to mitigate this issue.
Understanding the impact of JavaScript and how to manage its execution efficiently is vital for optimization. Below are several strategies that can help you minimize JavaScript execution time and improve page load speeds.
Optimize Script Loading
- Defer Non-Critical Scripts: Use the defer attribute in the
<script>
tag to delay non-essential scripts until after the initial page load. - Async Loading: Use the async attribute to load scripts asynchronously, which prevents them from blocking the rendering process.
- Minify JavaScript: Minification removes unnecessary characters like spaces and comments, reducing file sizes and enhancing load time.
Code Optimization Techniques
- Reduce Complex DOM Manipulations: Minimize the number of changes to the DOM to avoid layout recalculations and reflows, which can be time-consuming.
- Efficient Loops: Optimize loops, especially when processing large datasets, to ensure they don’t perform excessive computations.
- Limit Use of Heavy Libraries: Consider using smaller, more focused libraries rather than large, all-encompassing ones.
By using a combination of deferred loading, asynchronous script execution, and optimized code, JavaScript can be a more efficient contributor to page performance rather than a bottleneck.
Analyze and Monitor JavaScript Performance
Tool | Description |
---|---|
Chrome DevTools | Allows you to profile JavaScript execution and track performance bottlenecks directly within the browser. |
Lighthouse | A performance auditing tool that provides actionable insights into JavaScript performance improvements. |
Webpack Bundle Analyzer | Helps visualize the size of JavaScript bundles and identify large dependencies that can be optimized or eliminated. |
Minimizing Resources that Block Page Rendering
In modern web development, the faster a page can display meaningful content, the better the user experience. One common obstacle to fast rendering is the presence of render-blocking resources, which delay the display of content until the resource is fully loaded. These resources typically include CSS, JavaScript, and web fonts, which can be loaded synchronously by default, blocking the rest of the page from being rendered until they are completely fetched and parsed.
To address this, developers can use several techniques to optimize the loading order of these resources. By deferring or asynchronously loading non-critical resources, the browser can prioritize rendering the page's essential content without waiting for less important files to load first.
Effective Methods for Reducing Render-Blocking Resources
- Defer JavaScript Loading: Use the "defer" or "async" attributes for external script tags to prevent them from blocking the rendering process.
- Inline Critical CSS: Include only the essential CSS directly in the HTML head, allowing the page to load without waiting for the entire stylesheet.
- Preload Key Resources: Use the
rel="preload"
attribute to load critical resources, such as fonts or images, before other resources. - Lazy Load Non-Essential Images: Delay the loading of images that are not in the viewport until the user scrolls to them.
Common Issues with Blocking Resources
External CSS files are often a major culprit for blocking rendering. By making them external and synchronous, the browser needs to fully download and parse them before rendering the page. This can cause significant delays, especially if the CSS is large or hosted on slow servers.
Table of Best Practices
Technique | Effect | How to Implement |
---|---|---|
Defer JavaScript | Prevents JavaScript from blocking page rendering | Use defer or async attributes in script tags |
Inline Critical CSS | Loads essential CSS immediately for faster rendering | Embed critical CSS directly in the <head> of the page |
Lazy Loading | Delays loading of images and media to speed up initial render | Implement the loading="lazy" attribute for images |
Image Optimization: Balancing File Size and Quality for Faster Load Times
When optimizing images for faster web performance, it's crucial to find the right balance between file size and image quality. While smaller images contribute to faster download speeds, overly compressing them can result in noticeable loss of detail. The challenge lies in minimizing file size without sacrificing visual clarity, ensuring the best experience for users across different devices and network conditions.
Various methods and tools can be used to achieve this balance, from choosing the appropriate file formats to applying compression techniques that preserve the image's appearance. Understanding these methods will help you make informed decisions about how to optimize images for speed without compromising user experience.
Key Considerations for Optimizing Images
- Image Format: Selecting the right format (JPEG, PNG, WebP, etc.) significantly impacts both file size and quality.
- Compression Techniques: Lossy vs. lossless compression can greatly influence image clarity and file size.
- Resolution and Dimensions: Resizing images to the correct dimensions can reduce unnecessary file weight.
"Reducing image file size is not just about compression but also choosing the right format for the context. WebP often offers better quality at smaller file sizes compared to JPEG or PNG."
Recommended Image Compression Settings
Image Format | Compression Type | Best Use Case |
---|---|---|
JPEG | Lossy | Photographic images with gradients |
PNG | Lossless | Images with transparency or sharp edges |
WebP | Lossy and Lossless | Modern browsers, offering a good balance of size and quality |
Steps for Image Optimization
- Choose the Right Format: Assess the image content and select an optimal format.
- Resize Appropriately: Adjust the image dimensions based on how it will be displayed on the site.
- Apply Compression: Use tools to compress images without significant quality loss.
- Implement Lazy Loading: Load images only when they are needed on the user's viewport.
Improving Load Time Through Efficient Browser Caching
When optimizing the loading performance of a website, leveraging browser caching is one of the most effective techniques. By instructing the browser to store certain resources locally, websites can reduce redundant network requests and speed up page rendering. Cached content such as images, JavaScript, and CSS files do not need to be re-downloaded every time a user revisits a page, leading to faster load times and a more efficient user experience.
Effective use of caching can significantly reduce latency, especially for repeat visitors. The browser caches static assets based on certain cache control rules set by the server. These rules ensure that resources are stored for an appropriate amount of time, preventing unnecessary requests for unchanged files.
Strategies for Browser Caching Optimization
- Set Cache Expiration Headers: Properly configure cache expiration times to specify how long files should be stored locally before they need to be revalidated with the server.
- Use ETag or Last-Modified Headers: These headers enable the browser to compare cached content with the server’s version and determine if it has changed.
- Leverage Cache-Control Directives: Use directives like "public," "private," and "max-age" to control caching behavior for both static and dynamic resources.
Important: Proper cache management is crucial to avoid serving outdated content to users, which could lead to broken functionality or visual inconsistencies.
Example Cache-Control Header Configuration
Header | Purpose |
---|---|
Cache-Control: max-age=3600 | Caches the file for 1 hour (3600 seconds) |
Cache-Control: no-cache | Forces the browser to revalidate with the server before using the cached file |
Cache-Control: public | Indicates that the resource can be cached by any intermediate cache, like CDNs |
Improving Server Response Time with Proper Backend Configuration
Effective backend configuration plays a crucial role in reducing server response times and enhancing the overall performance of a web application. Optimizing server configurations ensures that requests are handled as efficiently as possible, minimizing delays caused by resource bottlenecks. This involves fine-tuning both the server hardware and the software environment to meet specific performance needs.
By properly configuring various aspects of the backend, developers can significantly improve the speed at which the server processes requests. This can be achieved through adjustments to caching, database queries, server software, and network settings, among other factors. Below are key areas to focus on for improving server performance:
- Database Optimization: Ensuring that database queries are efficient and indexes are properly utilized can drastically reduce the time needed to retrieve data.
- Connection Management: Proper management of server connections, such as using persistent connections and limiting the number of concurrent connections, helps in reducing the server load.
- Resource Allocation: Allocating enough memory, CPU, and disk I/O resources ensures the server can handle heavy loads without bottlenecks.
Proper server configuration is essential for reducing latency and ensuring optimal application performance, especially during traffic spikes.
Techniques for Optimizing Server Response
- Use of Caching: Leverage caching mechanisms such as Memcached or Redis to store frequently accessed data in memory, reducing database load and speeding up response times.
- Content Delivery Networks (CDNs): Offload static content to CDNs to reduce server workload and speed up content delivery across different regions.
- Optimize Web Server Configuration: Fine-tune web server settings (e.g., Nginx or Apache) for handling concurrent connections and ensuring low-latency processing.
Key Configuration Settings
Setting | Benefit |
---|---|
MaxConnections | Limits the number of simultaneous connections, preventing server overload during high traffic. |
Timeouts | Reducing timeouts for slow requests to free up resources and avoid blocking critical processes. |
Compression (GZIP) | Reduces the size of data sent between server and client, improving transfer speed. |
Implementing Lazy Loading for Non-Essential Resources
Lazy loading is a technique that helps to optimize page load time by deferring the loading of non-critical resources until they are actually needed. This approach is particularly useful for websites with heavy media content, large images, or third-party scripts that do not impact the initial rendering of the page. By loading resources only when they are in view or needed by the user, the overall performance improves, resulting in a faster page load time and better user experience.
For instance, images or videos that are placed lower in the page and are outside the initial viewport can be loaded only when the user scrolls down. Similarly, non-essential JavaScript and CSS files can be loaded after the main content has already been rendered, reducing the initial download size and improving the perceived speed of the website.
Benefits of Lazy Loading Non-Essential Resources
- Reduced initial page load time: By postponing the loading of non-essential resources, the page renders faster, leading to improved user experience.
- Lower bandwidth usage: Only the necessary resources are loaded initially, saving bandwidth for users with limited data plans.
- Improved SEO: Search engines can index content more efficiently, as lazy loading ensures that critical content is prioritized.
How to Implement Lazy Loading
- Images: Use the loading="lazy" attribute for images in HTML, or utilize JavaScript libraries like Intersection Observer for more control.
- JavaScript: Implement asynchronous loading of non-essential scripts using the
async
ordefer
attributes in script tags. - CSS: Load non-critical CSS files after the page has loaded using JavaScript to dynamically inject them into the DOM.
"Lazy loading allows resources to be loaded only when required, providing a better balance between performance and functionality."
Considerations
Factor | Impact |
---|---|
Browser Support | Modern browsers support native lazy loading for images, but some older browsers may require fallback solutions. |
SEO Considerations | Ensure critical resources are not lazy-loaded, as this could hinder search engine crawlers from fully indexing the page. |
Optimizing Global Performance with Content Delivery Networks (CDNs)
Content Delivery Networks (CDNs) are essential tools for improving the speed and efficiency of web applications, especially when targeting users in diverse geographical locations. By leveraging a distributed network of servers, CDNs store cached content at various points around the world, ensuring faster delivery to end-users by reducing latency and improving load times.
When applied effectively, CDNs enhance both static and dynamic content delivery, making them invaluable for global performance optimization. The use of these networks is crucial in reducing the load on origin servers, improving site availability, and providing better user experiences, regardless of user location.
Key Advantages of Using CDNs for Global Performance
- Reduced Latency: CDNs minimize the distance between users and the server, reducing the time it takes to retrieve data and improving the speed of content delivery.
- Enhanced Site Availability: Multiple servers in different regions ensure high availability even if one server goes down or experiences issues.
- Improved Load Times: Content is delivered from the closest server, decreasing loading times significantly, especially for users located far from the main server.
- Efficient Bandwidth Usage: By caching static content, CDNs offload traffic from origin servers, optimizing bandwidth consumption and reducing costs.
Considerations for CDN Implementation
- Content Caching: Make sure to configure the appropriate caching rules to ensure dynamic content is efficiently handled, and only static content is cached unless necessary.
- Geographical Coverage: Choose a CDN provider with a global presence to guarantee content delivery across all user regions, reducing the likelihood of slow load times.
- SSL/TLS Integration: Ensure that the CDN supports secure connections with proper SSL/TLS encryption, especially when handling sensitive data.
- Performance Monitoring: Continuously monitor the CDN's performance to identify potential issues with content delivery or server health.
Performance Metrics to Track
Metric | Description |
---|---|
Time to First Byte (TTFB) | Measures the time from the request until the first byte of data is received from the server. |
Latency | Time taken for a request to travel from the user to the server and back. |
Cache Hit Ratio | Percentage of content delivered from the CDN cache instead of the origin server. |
Throughput | Amount of data successfully delivered by the CDN in a given period. |
Leveraging a Content Delivery Network is one of the most effective strategies for ensuring that web applications maintain optimal performance and availability across the globe.
How to Regularly Track and Assess Website Performance
Maintaining optimal website performance is crucial to ensure user satisfaction and search engine ranking. Regular monitoring and audits are essential to identify performance bottlenecks and address them promptly. By adopting the right tools and techniques, you can keep your website running smoothly and efficiently over time.
To effectively monitor and assess performance, it's important to utilize automated tools that provide consistent insights into the website's health. These tools allow you to track critical metrics, such as load times, page rendering, and resource usage, which are key indicators of performance. Regularly performing audits ensures that you stay ahead of potential issues and optimize the user experience.
Key Methods to Track and Audit Website Performance
- Utilize Performance Monitoring Tools: Tools like Google Lighthouse, WebPageTest, and GTmetrix allow you to run audits on specific pages and measure various performance aspects.
- Set Up Real User Monitoring (RUM): Implement RUM to capture data on how real users experience the site, providing insight into actual performance rather than synthetic metrics.
- Measure Core Web Vitals: Focus on metrics like Largest Contentful Paint (LCP), First Input Delay (FID), and Cumulative Layout Shift (CLS) to monitor user-centric performance.
Steps for Regular Auditing
- Run Regular Audits: Schedule automated audits at regular intervals to track changes in performance over time.
- Analyze Results: Review audit results to identify areas of improvement, focusing on high-priority issues like slow page load times and inefficient resources.
- Implement Improvements: Based on audit findings, prioritize fixes, such as optimizing images, minifying scripts, or leveraging browser caching.
Regular auditing and monitoring ensure that performance issues are identified before they negatively affect user experience and SEO rankings.
Sample Performance Metrics
Metric | Description | Optimal Range |
---|---|---|
LCP (Largest Contentful Paint) | Time taken to render the largest visible content element. | Under 2.5 seconds |
FID (First Input Delay) | Time between the first user interaction and the browser's response. | Under 100 milliseconds |
CLS (Cumulative Layout Shift) | Measure of unexpected layout shifts during page load. | Under 0.1 |