Route Optimization in R
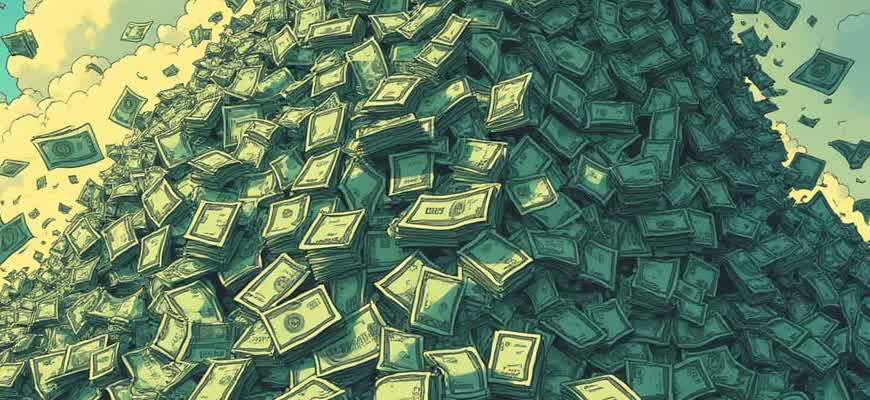
Route optimization involves finding the most efficient path for a given set of destinations, aiming to reduce costs such as travel time or fuel consumption. In R, this process can be achieved using several specialized packages and algorithms that tackle the Traveling Salesman Problem (TSP) and Vehicle Routing Problem (VRP).
To implement route optimization in R, a few essential steps need to be followed:
- Data preparation: Gather coordinates and other relevant data for the locations involved.
- Model selection: Choose the right optimization model based on the problem type (TSP, VRP, etc.).
- Algorithm application: Use R packages like TSP, vrp, or igraph to implement the chosen model.
Important: Understanding the problem type is crucial for selecting the correct optimization method. For example, TSP is typically used for a single vehicle, while VRP involves multiple vehicles with various constraints.
R provides powerful tools to handle these optimizations, offering flexibility in defining distance matrices and applying heuristic methods for large-scale problems.
Package | Use Case | Key Functions |
---|---|---|
TSP | Solving the Traveling Salesman Problem | solve_TSP(), as.dist() |
vrp | Vehicle Routing Problem with constraints | vrp(), plot_vrp() |
igraph | Graph-based optimization | shortest_paths(), igraph() |
Setting Up R for Route Optimization: Required Packages and Libraries
Before diving into the specifics of route optimization in R, it’s important to ensure that the necessary environment is properly configured. The functionality of R for solving optimization problems relies on a variety of specialized libraries that facilitate tasks such as distance calculation, route generation, and problem-solving using algorithms like the Traveling Salesman Problem (TSP). These libraries allow R to handle large datasets and apply different optimization techniques efficiently.
In the next sections, we’ll focus on the essential libraries that should be installed and loaded to begin working on route optimization. Whether you're aiming to calculate the shortest path, analyze traffic data, or visualize the routes, having the right set of tools is critical for success.
Key Libraries for Route Optimization
Below is a list of the most widely used packages in R for route optimization tasks:
- Geosphere: Provides functions for geospatial calculations, such as computing distances between geographic points and calculating great-circle distances.
- TSP: Implements algorithms for solving the Traveling Salesman Problem (TSP), including exact and heuristic methods.
- gDistance: Part of the raster package, it offers functions for calculating distances between raster cells and vector points.
- igraph: Offers tools for creating, manipulating, and analyzing graphs, including functionalities for finding shortest paths.
- Leaflet: A powerful tool for interactive map visualization, allowing the representation of routes on maps.
Installing Required Packages
To get started with route optimization in R, you need to install these libraries. Below is an example of how to install and load them:
install.packages("Geosphere") install.packages("TSP") install.packages("igraph") install.packages("leaflet")
After installation, you can load the libraries into your R session:
library(Geosphere) library(TSP) library(igraph) library(leaflet)
Example of Installation Table
Package Name | Purpose |
---|---|
Geosphere | Geospatial distance and spatial analysis. |
TSP | Solving the Traveling Salesman Problem. |
igraph | Graph-based route analysis and shortest path calculation. |
Leaflet | Visualization of routes on interactive maps. |
Note: It’s crucial to install all required libraries before beginning to implement any route optimization models. These packages provide the tools needed to process, analyze, and visualize geographic data effectively.
Choosing the Right Algorithm for Your Route Optimization Problem
When it comes to solving route optimization problems in R, selecting the most appropriate algorithm is crucial for achieving both efficiency and accuracy. The choice of algorithm depends on factors such as the complexity of the problem, the nature of the data, and the performance requirements of the solution. For example, a simple problem with a small dataset may benefit from brute-force methods, while larger, more complex datasets may require heuristic or metaheuristic algorithms to provide optimal or near-optimal solutions in a reasonable time frame.
Understanding the different types of algorithms available can help guide your decision-making process. In this context, it's essential to evaluate both exact and approximate methods to determine which one aligns best with your project's specific needs.
Types of Algorithms
- Exact Algorithms: These guarantee an optimal solution but may require significant computational resources, especially for large problems. Examples include:
- Integer Programming (IP): Uses mathematical programming techniques to find the optimal route.
- Branch and Bound: An algorithm that systematically explores the solution space and prunes suboptimal solutions.
- Heuristic Algorithms: These algorithms provide good solutions in a reasonable amount of time but do not guarantee the optimal result. They are suitable for larger, more complex problems. Examples include:
- Nearest Neighbor: A greedy approach that selects the closest point at each step.
- Greedy Algorithms: Simplifies the problem by making the best choice at each step without considering the global optimum.
- Metaheuristic Algorithms: These algorithms, such as Genetic Algorithms, Simulated Annealing, and Ant Colony Optimization, aim to explore the solution space more effectively and find high-quality solutions, especially for large-scale, complex problems.
- Genetic Algorithm: Mimics the process of natural selection to evolve better solutions over time.
- Simulated Annealing: Inspired by the annealing process in metallurgy, it finds solutions by exploring the solution space and gradually reducing the temperature (search space).
Factors to Consider
Factor | Description |
---|---|
Problem Size | Smaller problems may benefit from exact algorithms, while larger problems may require heuristic or metaheuristic methods. |
Solution Accuracy | If an exact solution is necessary, exact algorithms should be considered, but if a near-optimal solution is acceptable, heuristics or metaheuristics can be effective. |
Computational Resources | Exact algorithms may demand significant computational power, so it’s important to balance resources with the required solution quality. |
"The key to selecting the right algorithm lies in understanding the trade-offs between solution quality, computational effort, and problem size."
Solving the TSP Problem with R
The Traveling Salesman Problem (TSP) is a classic optimization issue where the goal is to find the shortest route that allows a salesman to visit each city once and return to the starting point. This problem has many practical applications, such as logistics and route planning. In R, various approaches, including brute force, dynamic programming, and metaheuristics like genetic algorithms, can be used to solve TSP.
To implement TSP in R, we can leverage packages such as `TSP` or `gtools`. These provide built-in functions to help in finding efficient solutions, even for larger instances. Let's explore a basic approach using the `TSP` package to demonstrate solving this problem efficiently.
Steps to Implement TSP in R
- Install the necessary package:
install.packages("TSP")
- Load the package:
library(TSP)
- Create a distance matrix representing the cities and the distances between them.
- Use the built-in `solve_TSP` function to find the optimal route.
Example: Solving TSP with Random Cities
The following is an example of how to implement a TSP solver in R using random city coordinates:
# Load necessary libraries library(TSP) # Generate a random distance matrix set.seed(123) cities <- matrix(runif(10*2), ncol=2) dist_matrix <- dist(cities) # Create TSP instance tsp_instance <- TSP(dist_matrix) # Solve the problem using the nearest neighbor heuristic route <- solve_TSP(tsp_instance, method="nearest_insertion") route
Note: This method uses the nearest neighbor heuristic, which is fast but may not always provide the optimal solution.
Understanding the Output
City | Position |
---|---|
1 | (0.381, 0.922) |
2 | (0.272, 0.117) |
3 | (0.802, 0.648) |
4 | (0.631, 0.782) |
5 | (0.689, 0.245) |
The `solve_TSP` function will return a sequence of cities that forms the optimal or near-optimal path, minimizing the total travel distance. The heuristic method used here is a balance between speed and solution accuracy.
Visualizing Route Solutions in R: Best Practices
In route optimization, the visualization of solutions is a critical step for understanding and interpreting the results. Using R for this purpose can provide powerful graphical representations of routes, helping to identify inefficiencies, bottlenecks, and opportunities for improvement. By leveraging libraries such as ggplot2, leaflet, and plotly, users can create intuitive and interactive maps that clearly demonstrate the optimized paths.
Effective visualization not only aids in decision-making but also in presenting results to stakeholders. Proper design and customization of these visualizations enhance clarity, making complex routing data more accessible. In this guide, we'll outline key strategies for visualizing optimized routes in R using best practices to ensure maximum interpretability and usefulness.
Key Considerations for Route Visualization
- Clarity of Data Representation: Keep visualizations clean and straightforward. Avoid cluttering the map with unnecessary elements or excessive details.
- Interactive Features: Use interactive tools, such as leaflet or plotly, to allow users to zoom in, pan, and hover over different route elements.
- Color Coding: Use contrasting colors for different routes or waypoints to improve visibility and distinguish between multiple paths.
- Annotations: Annotate key points such as start, end, and critical decision points to provide additional context to the viewer.
Common Visualization Techniques
- Route Mapping with Leaflet: This package allows for creating interactive maps with customizable markers, polylines, and popups, which are essential for visualizing route data on geographical maps.
- Route Highlighting with ggplot2: A great tool for plotting routes on static maps. ggplot2 provides flexibility for custom plotting, including adjusting line thickness, color, and transparency.
- Network Diagrams: Network-based visualizations help in understanding route connections and overall structure, particularly useful in logistics and transportation planning.
Important Considerations for Enhancing Route Visualizations
Key Tip: Always consider the scale of the map and data. Too large a scale may obscure details, while too small a scale may make it difficult to discern relevant route patterns.
Visualization Type | Best Use Case | Recommended Library |
---|---|---|
Interactive Map | Displaying real-time route optimization or interactive user interfaces | leaflet, plotly |
Static Map | Visualizing routes for static reports and presentations | ggplot2 |
Network Diagram | Illustrating complex routing systems with multiple connections | igraph, ggplot2 |