Arduino Traffic Light with Push Button Code
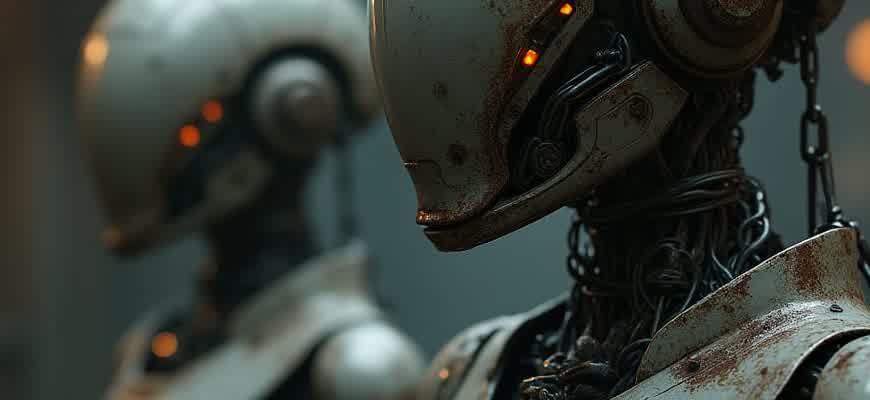
Creating a traffic light system with Arduino involves using various electronic components to control a series of LEDs simulating traffic signals. The addition of a push button enables manual control of the traffic light sequence, which adds an interactive element to the project. Below is a breakdown of the components and basic structure for this type of project.
- Arduino board (e.g., Uno)
- Red, Yellow, and Green LEDs
- Push button
- Resistors (220Ω for LEDs, 10kΩ for button)
- Jumper wires and breadboard
In the following steps, we will wire up the components and write the code to manage the traffic light cycles based on the button input.
Important: Ensure proper grounding for the push button to avoid unexpected behavior in the circuit.
- Connect the LEDs to the designated pins on the Arduino, typically using digital pins for control.
- Wire the push button to an available digital input pin and configure the circuit to detect button presses.
- In the code, set up the LED pins as outputs and the push button pin as an input.
Component | Pin Number |
---|---|
Red LED | Pin 2 |
Yellow LED | Pin 3 |
Green LED | Pin 4 |
Push Button | Pin 5 |
Setting Up the Circuit for Traffic Light and Push Button with Arduino
To create a working traffic light simulation with an Arduino, you need to connect several components. The traffic light consists of three LEDs representing the red, yellow, and green signals. Additionally, a push button allows the user to control the switching between the light sequences. The setup is simple but requires careful attention to ensure the correct wiring and functionality.
Here is how to wire the Arduino, LEDs, and push button to begin building the circuit:
Wiring Components
- LEDs: Three LEDs (Red, Yellow, Green) need to be connected to three digital pins on the Arduino (e.g., pins 3, 4, and 5). Each LED should have a current-limiting resistor (220Ω to 330Ω) connected to the long leg (anode).
- Push Button: The push button should be connected between the ground (GND) and a digital pin (e.g., pin 2) on the Arduino. Use a pull-up resistor to prevent floating input.
- Resistors: For the LEDs, resistors are essential to limit current and avoid damaging the components.
Step-by-Step Circuit Connection
- Connect the red LED to pin 3, the yellow LED to pin 4, and the green LED to pin 5.
- Place the short leg (cathode) of each LED to the ground (GND).
- Wire the push button to pin 2 and connect one end to ground.
- Ensure a pull-up resistor (10kΩ) is connected between the input pin (pin 2) and the VCC to stabilize the signal.
- Test the circuit by uploading the code to the Arduino and checking that each light changes with the push of the button.
Note: Ensure you use the correct resistors to prevent overloading your components and to ensure the longevity of your circuit.
Component Overview
Component | Connection | Purpose |
---|---|---|
Red LED | Pin 3 | Displays the red signal |
Yellow LED | Pin 4 | Displays the yellow signal |
Green LED | Pin 5 | Displays the green signal |
Push Button | Pin 2 | Controls the light transitions |
Understanding the Push Button Mechanism in the Traffic Light System
In traffic light systems controlled by Arduino, the push button serves as an essential component for manually overriding the automatic light cycle. It enables users to interact with the system, often to request a change in traffic light state. This simple input device is integrated into the circuit to signal the microcontroller, which in turn controls the traffic light sequence based on the button's state.
The button typically operates through a simple digital input mechanism. When pressed, it sends a LOW signal to the microcontroller, triggering an event in the program that alters the current state of the traffic light. This interaction can be used to pause the regular cycle and trigger a specific light change, like switching to green for pedestrians or vehicles.
Working Principle of the Push Button
- Button State Change: When the button is pressed, it changes the state of the input from HIGH to LOW (or vice versa, depending on the wiring configuration).
- Signal Processing: The microcontroller reads this input signal and responds by altering the flow of traffic lights accordingly.
- Debouncing: To prevent multiple triggers from one press, a debouncing technique is often implemented in the code, ensuring that only a single event is detected per press.
Steps in the Push Button Interaction
- When the button is pressed, the state of the button input pin changes.
- The microcontroller detects this change and triggers the traffic light sequence based on the logic coded in the system.
- After a predetermined time, the system reverts to its original cycle or waits for another button press.
Note: It is crucial to account for any noise or bouncing signals in the input pin. This is typically handled by adding a short delay or using hardware debouncing techniques.
Traffic Light Control Table
Traffic Light | Normal State | On Button Press |
---|---|---|
Red | On for vehicles, off for pedestrians | May remain red or switch to pedestrian mode |
Green | On for vehicles | May switch to red depending on system logic |
Yellow | On for vehicles for a short duration | May stay on for safety or revert to red |
Writing the Code to Control Traffic Light Timings Based on Button Input
When building a traffic light system with Arduino, it's important to control the timing of the traffic light phases dynamically. A push button can serve as a way to modify these timings. For instance, pressing the button could switch between different time intervals for red, yellow, and green lights. The goal is to write code that reads button presses and adjusts the timing accordingly. This introduces an interactive aspect to the traffic light system.
The button input will be mapped to a certain function that changes the duration of each light phase. By using simple logic in the code, the program can monitor the state of the button and adjust the light timings in real-time. This enables the system to react to user input without needing to restart or reset the Arduino board.
Steps to Implement Button-Controlled Timing
- Set up the button to read its state (pressed or not).
- Define different time intervals for each light color (red, yellow, green).
- Map the button press to a function that modifies these intervals.
- Use conditional statements to cycle through different light timings when the button is pressed.
Code Logic
- Initialize the button input pin and set the traffic light pins as output.
- Use the digitalRead() function to detect button presses.
- Assign different time durations to the light phases based on the button state.
- Employ the delay() function to control the time each light stays on.
- Cycle through the red, yellow, and green lights as per the updated timings.
Important: Ensure that the button is debounced to prevent multiple readings during a single press. Use a small delay or a software debounce method to avoid inaccurate timing.
Sample Timing Table
Light Color | Normal Duration | Button Pressed Duration |
---|---|---|
Red | 5000 ms | 7000 ms |
Yellow | 2000 ms | 3000 ms |
Green | 4000 ms | 6000 ms |
Integrating Debouncing Logic for Reliable Push Button Functionality
When working with an Arduino-based traffic light system, the push button is often used to trigger events, such as switching between different light modes. However, mechanical push buttons can introduce noise into the system, causing multiple unintended presses to be registered when only a single press occurs. This issue is called "switch bouncing." Properly managing this behavior through debouncing techniques ensures that the button press is registered only once, preventing errors in the system’s operation.
Debouncing logic helps to stabilize the signal from the button, filtering out the noise that results from the button's mechanical contacts bouncing. The simplest method for debouncing is by using a software delay, which introduces a brief pause after each press to give the switch time to settle. More advanced methods, such as state machines or external components like capacitors, can also be employed for more robust solutions. Below is a breakdown of how to implement effective debouncing in your traffic light project.
Debouncing Techniques
- Software Delay: A simple delay after detecting a press prevents multiple triggers.
- State Machine: A state machine can track the button state, ensuring that the press is valid and stable before executing actions.
- External Components: Capacitors or resistors can be used to smooth out the signal and eliminate noise at the hardware level.
Example Code Snippet
const int buttonPin = 2; // Push button pin const int ledPin = 13; // LED pin unsigned long lastPress = 0; // Time of last button press unsigned long debounceDelay = 50; // Debounce delay in milliseconds void setup() { pinMode(buttonPin, INPUT); pinMode(ledPin, OUTPUT); } void loop() { int buttonState = digitalRead(buttonPin); // Check if the button state has changed and debounce if (buttonState == HIGH && (millis() - lastPress) > debounceDelay) { digitalWrite(ledPin, !digitalRead(ledPin)); // Toggle LED lastPress = millis(); // Update the last press time } }
Table of Debouncing Methods
Method | Description | Pros | Cons |
---|---|---|---|
Software Delay | Introduce a short delay after each button press to avoid multiple detections. | Simple to implement, no extra components needed. | May introduce slight input lag; not suitable for fast or high-frequency presses. |
State Machine | Tracks button states across transitions and registers a press only when it is stable. | More reliable, no additional hardware needed. | More complex code structure, requires more resources. |
External Components | Using capacitors or resistors to smooth the button's signal. | Hardware solution, very reliable. | Requires additional components and potentially more space on the board. |
Debouncing is crucial for any project that involves push buttons to ensure that the button press is accurately detected and processed without errors caused by mechanical bouncing.
Adjusting Traffic Light Cycle Duration for Real-World Scenarios
When designing a traffic light system, it is crucial to adjust the duration of each light phase based on real-world factors. The timing of red, yellow, and green lights should reflect traffic flow, pedestrian crossings, and the time of day. A simple static cycle can lead to inefficiencies, such as unnecessary wait times or unsafe conditions for both drivers and pedestrians. To achieve optimal traffic management, it’s important to tailor the light cycle duration to these changing conditions.
Various methods can be employed to dynamically adjust the traffic light cycle. A basic approach is incorporating traffic sensors or push buttons for pedestrian crossings, allowing the system to modify the light durations accordingly. More advanced systems might include algorithms that consider traffic density, peak hours, and the current weather, adjusting the signal timing in real-time.
Methods for Adjusting Traffic Light Timing
- Traffic Sensors: Automatically adjust the light cycle based on the number of vehicles detected at an intersection.
- Push Buttons: Pedestrians can press a button to extend the green light or change the cycle for safe crossing.
- Time-of-Day Adjustments: Longer green light durations during peak traffic hours, shorter during off-peak hours.
Implementation Considerations
It is important to ensure that any system designed to modify the traffic light cycle in real-time does not lead to unintended delays or hazards, especially during high-traffic periods.
- Establish baseline durations: The initial cycle timing can be set for typical traffic patterns.
- Integrate sensors and push buttons: Allow for adaptive timing based on vehicle and pedestrian presence.
- Monitor performance: Regularly assess how the adjusted cycle improves or hinders traffic flow.
Sample Cycle Duration Adjustments
Time of Day | Green Light Duration | Red Light Duration |
---|---|---|
Morning (7 AM - 9 AM) | 60 seconds | 30 seconds |
Midday (12 PM - 2 PM) | 45 seconds | 30 seconds |
Evening (5 PM - 7 PM) | 90 seconds | 45 seconds |
Debugging Common Issues When Building the Arduino Traffic Light System
When building a traffic light system with Arduino and a push button, there are several common issues that may arise during development. Troubleshooting is an essential part of the process, as it ensures the system works as intended. Most problems occur due to wiring issues, incorrect code logic, or faulty components. In this guide, we’ll address some typical issues and solutions that will help you debug effectively.
Understanding where the problem lies is the first step in resolving any issue. Whether it's the traffic lights not switching, the button failing to work, or the LEDs not lighting up, each problem has its own set of solutions. Let’s take a closer look at some common debugging scenarios.
Common Issues and Solutions
- Button Not Responding: If the push button doesn’t register inputs, it might be due to improper wiring or a missing pull-down resistor. Ensure that the button is connected to both the Arduino pin and ground, with the appropriate resistor in place.
- Lights Sticking: If the lights stay on one color, check the logic in your code. It could be due to missing delays, incorrect conditions, or issues with the state transitions between red, yellow, and green.
- Wrong LED Behavior: If an LED doesn't light up or lights up unexpectedly, ensure the corresponding pin mode is set to OUTPUT and the wiring is correct. Double-check your connections and test the LED with a simple blink program.
Code Debugging Tips
- Check if all pin modes are set correctly in the setup() function.
- Verify that the delays in your code are appropriate for realistic traffic light timing.
- Make sure that the button state is read correctly using digitalRead() and debounce the button input to prevent false readings.
Table of Common Issues and Solutions
Issue | Possible Cause | Solution |
---|---|---|
Button not working | Incorrect wiring or missing pull-down resistor | Check wiring, add a pull-down resistor, or use internal pull-up resistor in code |
LEDs not responding | Incorrect pin mode or faulty wiring | Ensure pinMode() is set to OUTPUT, test LEDs with simple blink code |
Lights not switching | Logic error in code or missing delay | Check if conditions for switching states are correct, add delays between state changes |
Tip: Always test your hardware and code step-by-step, isolating the issues one at a time. Debugging can be more efficient if you focus on individual components rather than the entire system.
Simulating Traffic Light Functionality Without Physical Components
When designing traffic light systems with Arduino, it's common to use physical components such as LEDs and buttons. However, for development and testing purposes, it is possible to simulate the behavior of these components entirely through software. This can be accomplished using various simulation platforms that mimic the logic of the traffic light system, allowing developers to test code before interacting with actual hardware.
By simulating the traffic light system, developers can ensure that the timing of lights and the response to user inputs (like pressing a button) work correctly. This approach not only saves time but also provides a controlled environment for debugging and refining code without the need for expensive or bulky physical setups.
Steps to Simulate Traffic Light Behavior
- Choose a Simulation Platform: Select a software tool like Tinkercad or Proteus that allows Arduino code to be tested without physical components.
- Setup Virtual Components: Place virtual LEDs for red, yellow, and green lights, and create a virtual push button in the simulation environment.
- Write the Code: Implement the Arduino code that controls the LEDs and handles the button press logic, ensuring the correct sequence of lights.
- Test and Debug: Run the simulation to observe the behavior of the traffic light system, making adjustments as necessary.
Simulating a traffic light system can provide valuable insights into the system's logic and help identify potential issues before physical implementation.
Table of Components for Simulation
Component | Description |
---|---|
LED (Red, Yellow, Green) | Used to represent traffic lights in the simulation. |
Push Button | Simulates the user input for changing the light sequence. |
Arduino Board | Acts as the controller for the simulation, executing the code logic. |
By following these steps, you can effectively simulate and test traffic light behaviors before committing to physical hardware, making the development process smoother and more efficient.
Optimizing Traffic Light Control Code for Smooth Transitions
When designing an Arduino-based traffic light system, ensuring that the transitions between different light states are smooth and efficient is crucial. To achieve this, the code must be optimized to handle light changes without delay or flickering, while also allowing for user interaction through a push button. Proper sequencing, timing, and state management are essential for a functional and responsive system.
By refining the logic and structure of your code, you can minimize delays and improve the overall performance of the traffic light system. It’s important to consider factors such as timing accuracy, efficient use of loops, and the handling of button presses to prevent conflicts between the light transitions and user inputs.
Key Optimization Strategies
- Efficient use of delays: Instead of using long delay functions, try to implement non-blocking timing using the millis() function. This helps keep the system responsive without causing any freezing during the transitions.
- State management: Use a state machine approach to manage the light sequence. This allows for easy tracking of the current state and smooth transitions between the red, yellow, and green lights.
- Button debounce handling: Incorporate a debounce technique to avoid multiple reads from a single press, which can interfere with the traffic light cycle.
Sample Optimization Techniques
- Use millis() instead of delay() for time-based actions to improve responsiveness.
- Implement a state machine to keep track of the traffic light’s current state and ensure that each transition happens seamlessly.
- Apply button debounce logic using digitalRead() with a small delay to avoid multiple button presses being registered in a single cycle.
Tip: Combining non-blocking delays and a state machine approach is key to achieving smooth and efficient transitions in your traffic light system.
Example Timing Table
Light State | Duration (ms) |
---|---|
Red | 5000 |
Green | 5000 |
Yellow | 2000 |