Q-learning Algorithm Python
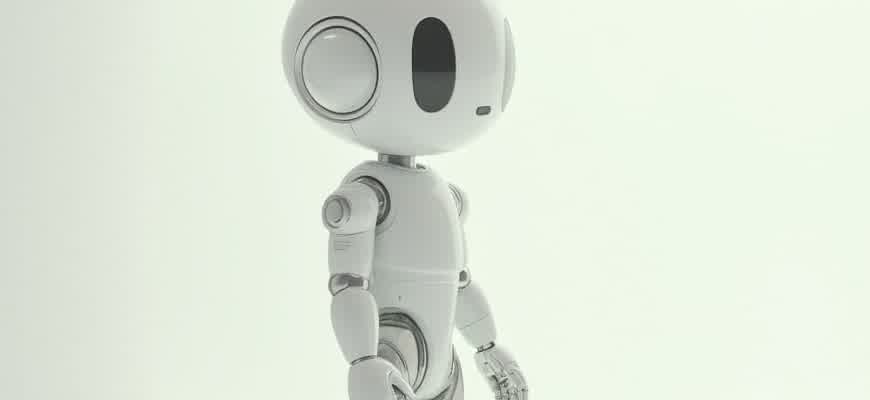
The Q-learning method is a type of reinforcement learning algorithm that enables an agent to learn how to make decisions by interacting with an environment. In simple terms, it helps the agent determine the best possible actions to maximize cumulative rewards over time. Python provides an excellent environment for implementing and experimenting with Q-learning due to its extensive libraries and simplicity.
To understand how Q-learning operates, let's first break down some key concepts:
- State (S): A representation of the current situation of the environment.
- Action (A): A possible move or decision an agent can make in a given state.
- Reward (R): The feedback received after taking an action in a given state, usually numeric.
- Q-value (Q): A function that estimates the total expected future reward for a given state-action pair.
"The Q-learning algorithm updates its Q-values iteratively based on the Bellman equation, aiming to find the optimal policy for decision-making."
In Python, the Q-learning algorithm can be implemented with simple logic using numpy arrays to store Q-values and iterating through states and actions. The algorithm follows these main steps:
- Initialize Q-table with zeros.
- For each episode, select an action based on the current policy (e.g., epsilon-greedy).
- Take the action, observe the reward and the new state.
- Update the Q-value using the Bellman equation:
Q(s, a) = Q(s, a) + α * [R + γ * max(Q(s', a')) - Q(s, a)]. - Repeat the process until the Q-values converge to optimal values.
Variable | Description |
---|---|
α | Learning rate, controls how much new information overrides old information. |
γ | Discount factor, determines the importance of future rewards. |
ε | Exploration rate, used in the epsilon-greedy strategy for action selection. |
Q-Learning Algorithm in Python: Practical Guide for Implementation
Q-learning is a model-free reinforcement learning algorithm that helps an agent learn how to achieve its goal by interacting with an environment. It works by updating a Q-table based on the rewards received after each action, allowing the agent to learn the optimal policy. In Python, implementing Q-learning is a straightforward process, with libraries such as NumPy and OpenAI's Gym making it easier to experiment and test various environments.
The key to the Q-learning algorithm is its ability to update the Q-values using the Bellman equation. This allows the agent to estimate the future rewards of its actions and adjust its strategy accordingly. Below is a guide for implementing Q-learning in Python, which covers setting up the environment, initializing the Q-table, and updating the values during training.
Steps to Implement Q-learning in Python
- Import necessary libraries: First, import libraries like NumPy for mathematical operations and OpenAI's Gym for simulation environments.
- Initialize Q-table: Create a Q-table that stores the expected future rewards for each state-action pair. Initialize the table with zeros.
- Choose an action: Use an epsilon-greedy strategy to balance exploration and exploitation. With probability epsilon, the agent will explore random actions.
- Update the Q-table: After performing an action, use the reward and the maximum future Q-value to update the Q-table according to the Bellman equation.
- Repeat the process: The agent continues interacting with the environment until it converges to the optimal policy.
Important: The epsilon value plays a crucial role in the agent's learning process. It controls the exploration-exploitation tradeoff, where higher epsilon values lead to more exploration and lower values focus on exploiting known information.
Sample Code
import numpy as np import gym # Initialize environment env = gym.make("Taxi-v3") # Initialize Q-table Q = np.zeros([env.observation_space.n, env.action_space.n]) # Hyperparameters learning_rate = 0.8 discount_factor = 0.95 epsilon = 0.2 # Training loop for episode in range(1000): state = env.reset() done = False while not done: if np.random.rand() < epsilon: action = env.action_space.sample() # Explore else: action = np.argmax(Q[state, :]) # Exploit next_state, reward, done, _ = env.step(action) # Update Q-value Q[state, action] = Q[state, action] + learning_rate * (reward + discount_factor * np.max(Q[next_state, :]) - Q[state, action]) state = next_state
Key Points to Remember
- Exploration vs. Exploitation: Always ensure a balance between exploring new actions and exploiting the best-known action. This is controlled by the epsilon parameter.
- Convergence: Q-learning can take a long time to converge, but the agent eventually learns the optimal policy.
- Discount Factor: The discount factor (gamma) determines how much future rewards are valued over immediate rewards. Higher values make the agent focus on long-term rewards.
Q-learning Performance Metrics
Metric | Description |
---|---|
Convergence Rate | Speed at which the Q-values approach their optimal values. |
Learning Efficiency | How efficiently the agent learns the optimal policy in terms of the number of episodes. |
Exploration Rate | Percentage of time the agent explores actions rather than exploiting known ones. |
How to Prepare Your Python Environment for Q-learning
Setting up a proper Python environment is essential for implementing Q-learning algorithms effectively. The first step is ensuring that you have the necessary packages installed, such as NumPy, TensorFlow or PyTorch (depending on your preference for deep learning integration), and gym for environment simulation. Additionally, creating a virtual environment helps isolate dependencies and avoid potential conflicts with other projects.
Once your environment is set up, the next step is configuring the workspace and verifying that all necessary modules are available for your Q-learning implementation. In this guide, we will break down the steps to configure your Python environment for a seamless Q-learning setup.
Step-by-Step Setup
- Install Python: Ensure you have Python 3.6 or higher installed. You can check the version with the command:
python --version
- Create a Virtual Environment: It's best to create a virtual environment to keep your dependencies isolated. Use the following commands:
python -m venv qlearning-env
source qlearning-env/bin/activate # On Linux/macOS
qlearning-env\Scripts\activate # On Windows
- Install Required Libraries: Once the virtual environment is active, install the necessary libraries for Q-learning:
pip install numpy gym tensorflow
Important Information
Make sure to keep your libraries up-to-date. Use the command pip install --upgrade package_name for updates.
Dependencies Overview
Library | Purpose |
---|---|
NumPy | For numerical operations and handling arrays |
Gym | Provides a variety of environments for reinforcement learning |
TensorFlow/PyTorch | For deep learning-based Q-learning implementations |
Implementing Q-learning from Scratch in Python: A Step-by-Step Approach
Q-learning is a type of reinforcement learning where an agent learns to make decisions by interacting with an environment. The agent learns the value of actions in different states and aims to maximize long-term rewards. Implementing this algorithm from scratch provides a deeper understanding of the underlying concepts, which is valuable for anyone looking to apply it in real-world problems.
In this guide, we will walk through the process of implementing Q-learning using Python. We will start with understanding the components required and then proceed step by step to create the learning agent. By the end of this tutorial, you will have a basic but functional Q-learning implementation to solve problems such as maze navigation or game-playing scenarios.
1. Define the Environment and Initialize Q-table
Before implementing the Q-learning algorithm, it's essential to define the environment in which the agent will operate. The environment typically consists of states, actions, and rewards. We initialize a Q-table, where each cell holds the expected future reward for taking a specific action in a given state.
- State: A representation of the current situation or position of the agent.
- Action: A possible move or decision the agent can make.
- Reward: The feedback given after the agent performs an action in a given state.
The Q-table is initialized with zeros, and as the agent explores the environment, these values will be updated.
2. Q-learning Algorithm Steps
- Initialize the Q-table: Set all the values of the Q-table to zero, as the agent has no prior knowledge of the environment.
- Choose an action: Using an epsilon-greedy strategy, the agent chooses either a random action or the action with the highest Q-value for the current state.
- Take the action and observe the reward: After performing the action, the agent receives a reward and transitions to a new state.
- Update the Q-value: Update the Q-value for the state-action pair using the formula:
Q(s, a) ← Q(s, a) + α [R(s, a) + γ max Q(s', a') − Q(s, a)]
Where:
- α: Learning rate (controls how much new information overrides old information).
- γ: Discount factor (how much future rewards are considered for current decisions).
- R(s, a): Immediate reward after taking action a in state s.
- max Q(s', a'): The maximum Q-value for the next state s'.
3. Example Q-table Structure
State | Action 1 | Action 2 | Action 3 |
---|---|---|---|
State 1 | 0.2 | 0.5 | 0.1 |
State 2 | 0.3 | 0.7 | 0.4 |
State 3 | 0.6 | 0.2 | 0.9 |
As the agent moves through the environment and updates the Q-table, the values will change, leading to better decisions over time. By iterating through the environment and refining the Q-values, the agent will eventually learn the optimal policy to maximize its long-term rewards.
Fine-Tuning Hyperparameters in Q-Learning for Optimal Results
In Q-learning, the performance of the algorithm heavily depends on the appropriate selection and fine-tuning of hyperparameters. These parameters influence the rate at which the model learns, the quality of the learned policy, and its ability to generalize across different states. The most critical hyperparameters include the learning rate, discount factor, and exploration-exploitation balance. Properly adjusting these values ensures faster convergence and more reliable results when training an agent in a reinforcement learning environment.
Fine-tuning involves iterating over different values for each hyperparameter to identify the optimal combination for the problem at hand. It is important to consider the specific characteristics of the environment and the goal of the agent when selecting these values. Below are key hyperparameters and their influence on the learning process:
Key Hyperparameters in Q-Learning
- Learning Rate (α): Controls the extent to which new information overrides previous knowledge. A high learning rate can lead to overshooting, while a low rate might slow down the learning process.
- Discount Factor (γ): Determines how much future rewards are valued over immediate ones. A higher γ places more weight on long-term rewards, encouraging the agent to explore more.
- Exploration Rate (ε): Balances the trade-off between exploration and exploitation. A higher ε promotes more exploration, which can help the agent discover better strategies in uncertain environments.
Steps for Fine-Tuning Q-Learning Hyperparameters
- Step 1: Initialize the parameters–Start with default or reasonable initial values for α, γ, and ε. For instance, α can be set to 0.1, γ to 0.9, and ε to 0.5.
- Step 2: Conduct experiments–Run multiple training sessions with varying values of hyperparameters. Track the performance of the agent in each case, focusing on the convergence rate and final policy quality.
- Step 3: Analyze results–Evaluate the performance by comparing reward accumulation, exploration behavior, and stability of the learned policy. A good balance between exploration and exploitation is crucial for robust learning.
Sample Hyperparameter Tuning Table
Learning Rate (α) | Discount Factor (γ) | Exploration Rate (ε) | Result |
---|---|---|---|
0.1 | 0.9 | 0.5 | Moderate convergence, balanced exploration-exploitation |
0.01 | 0.95 | 0.8 | Slower learning but potentially more stable long-term policy |
0.5 | 0.8 | 0.2 | Faster learning but prone to instability and suboptimal results |
Important: Hyperparameter tuning is an iterative process. It often requires a combination of manual tuning and automated methods like grid search or random search to achieve optimal performance.
Exploration vs. Exploitation in Q-learning Models
In Q-learning, one of the most crucial decisions during the learning process is how to balance exploration and exploitation. These two strategies dictate how the agent interacts with the environment. Exploration involves trying new actions to discover their potential rewards, while exploitation focuses on using known actions that maximize the current reward. A proper balance between these strategies is essential to ensure that the agent doesn't miss out on potentially better long-term rewards while also not constantly trying actions that may lead to suboptimal outcomes.
In practice, the balance between exploration and exploitation is often managed by using a parameter called the epsilon (ε) in the epsilon-greedy strategy. The epsilon-greedy method allows the agent to explore random actions with probability ε and exploit the best-known action with probability 1 - ε. This creates a trade-off, where a high ε encourages exploration, while a low ε favors exploitation.
Strategies for Balancing Exploration and Exploitation
- Exploration: Taking random actions, regardless of the current knowledge of the environment. This is useful when the agent has limited experience or when it is trying to discover better action policies.
- Exploitation: Using the actions that have historically provided the highest rewards. This leads to a more predictable and consistent strategy based on past learning.
Key Considerations
The balance between exploration and exploitation can significantly impact the overall performance of the Q-learning algorithm. Too much exploration can slow down the learning process, while too much exploitation can result in the agent getting stuck in suboptimal policies.
Adaptive Techniques
- Decay ε Over Time: One common approach is to gradually decrease ε as the agent learns more about the environment, moving towards a more exploitative strategy over time.
- Boltzmann Exploration: In this method, actions are chosen based on a probability distribution that favors actions with higher Q-values but still allows for exploration.
- Upper Confidence Bound (UCB): A more sophisticated approach that accounts for both the reward of an action and the uncertainty of its estimate, balancing exploration and exploitation dynamically.
Example Table: Epsilon-Greedy Strategy
Epsilon (ε) | Action | Resulting Behavior |
---|---|---|
High ε (e.g., 0.8) | Random action | Encourages exploration of unknown actions |
Low ε (e.g., 0.1) | Best-known action | Promotes exploitation of learned strategies |
Integrating Q-learning with OpenAI Gym for Reinforcement Learning Simulations
In reinforcement learning, the combination of Q-learning and OpenAI Gym provides a powerful framework for building intelligent agents capable of solving complex tasks. OpenAI Gym, with its wide array of environments, offers an excellent playground for training Q-learning models. By leveraging Gym's interface, Q-learning algorithms can be trained across various tasks ranging from simple environments like CartPole to more challenging ones like Atari games.
Q-learning is a model-free algorithm where an agent learns optimal policies through trial and error, using feedback from the environment in the form of rewards. By integrating it with Gym, the environment can simulate real-world scenarios, allowing the Q-learning agent to explore, learn, and refine its strategies. The process involves interacting with the environment, observing state transitions, and updating Q-values based on reward signals.
Steps for Integration
- Import Libraries – Start by importing necessary libraries, including Gym and the Q-learning framework.
- Create Environment – Use Gym to initialize the environment where the agent will operate.
- Define Q-learning Parameters – Set the learning rate, discount factor, and exploration strategy for the agent.
- Training Loop – Continuously update Q-values and let the agent explore the environment to improve performance.
Example: Table of Common Hyperparameters
Parameter | Description | Typical Value |
---|---|---|
Learning Rate (α) | Controls how much new information overrides old information | 0.1 - 0.9 |
Discount Factor (γ) | Determines the importance of future rewards | 0.9 - 0.99 |
Exploration Rate (ε) | Determines the probability of choosing a random action | 0.1 - 1.0 |
The integration of Q-learning with OpenAI Gym allows for easy experimentation with different environments, making it an ideal tool for researchers and developers in the field of reinforcement learning.
Identifying and Resolving Common Q-learning Issues in Python
When implementing the Q-learning algorithm in Python, developers often encounter specific errors that hinder the training process. Recognizing these errors early is essential for optimizing the algorithm's performance. Below are some common issues that arise in Q-learning implementations and strategies to resolve them.
Typical problems include incorrect initialization of Q-values, improper update of the Q-table, and issues related to the exploration-exploitation trade-off. Debugging these issues requires careful attention to the flow of the algorithm and understanding how Q-values should evolve over time.
Common Q-learning Errors and Solutions
- Improper Q-table Initialization: A common mistake is initializing the Q-table with inappropriate values (e.g., zeros for all states and actions). This can lead to biased or slow learning.
- Incorrect Q-value Update: Not applying the correct Q-value update formula can prevent convergence. Ensure that the Q-values are updated using the Bellman equation:
Q(state, action) = Q(state, action) + alpha * (reward + gamma * max(Q(next_state, all_actions)) - Q(state, action))
. - Exploration-Exploitation Issue: An unbalanced epsilon-greedy policy can result in too much exploration (random actions) or too much exploitation (choosing the best-known action). Tuning epsilon decay is important for the agent's learning efficiency.
Steps to Debug Q-learning Code
- Check Q-value Initialization: Start by reviewing how the Q-table is initialized. Set initial values close to zero or small random values.
- Verify Q-value Updates: Ensure the Bellman equation is correctly applied, with proper updates to the Q-values after each action.
- Monitor Exploration/Exploitation Balance: Adjust the epsilon value dynamically. Observe how the agent’s performance changes based on varying levels of exploration.
- Validate Reward Structure: Ensure that the reward function is well-defined and consistent with the task's objectives. Inconsistent rewards can confuse the learning process.
Tip: When debugging, print the Q-values after each update. This will help you track their evolution and verify if the Q-values are converging towards the optimal solution.
Debugging Example
Issue | Solution |
---|---|
Stagnant Q-values | Revisit the learning rate and ensure it’s not too small. A learning rate that is too low can slow down the learning process. |
Overfitting to Early States | Introduce a larger epsilon decay, so the agent can explore more diverse actions early in training. |
Missing State Transitions | Check that all state transitions are properly tracked in your environment. Missing transitions can lead to invalid Q-value updates. |
Visualizing the Outcomes of Q-Learning in Python
Visualizing the results of Q-learning is essential for understanding the agent's learning progress and evaluating its performance. Python provides several libraries that allow us to effectively display Q-values, actions, and rewards during the training process. By leveraging libraries such as Matplotlib, Seaborn, and Plotly, we can create insightful plots to illustrate the agent's behavior in various environments.
One common approach to visualize Q-learning results is through heatmaps, line plots, and 3D surface plots. These types of visualizations allow us to track how Q-values evolve over time and how the agent explores different states. Below are a few popular techniques for visualizing Q-learning results using Python libraries.
Popular Visualization Techniques
- Heatmaps are often used to display the Q-values of an agent for each state-action pair. They provide a clear representation of how the agent's knowledge evolves across the state space.
- Line plots can track the change in Q-values over time or during episodes, allowing us to observe the convergence of the learning process.
- 3D Surface Plots can be used to visualize the Q-values in environments with two continuous state variables, providing a more interactive and detailed view.
Example Visualization Using Matplotlib
A simple implementation of visualizing Q-values in a grid world can be done with the following code:
import matplotlib.pyplot as plt import numpy as np # Example grid of Q-values q_values = np.random.rand(5, 5) plt.imshow(q_values, cmap='coolwarm', interpolation='nearest') plt.colorbar() plt.title('Q-Values Heatmap') plt.show()
This code generates a heatmap that visualizes the Q-values in a 5x5 grid, helping to identify which states have been learned more effectively by the agent.
Performance Tracking
Tracking the agent's cumulative reward over time can help determine whether the Q-learning algorithm is performing well. The following is a basic implementation for visualizing the reward trend across episodes.
rewards = [1, 2, 3, 4, 5] # Example cumulative rewards over episodes plt.plot(rewards) plt.xlabel('Episodes') plt.ylabel('Cumulative Reward') plt.title('Reward Progression') plt.show()
Creating Q-Table Summary
A table can be used to summarize the final Q-values after training. The table format makes it easy to compare values across different states and actions.
State | Action 1 | Action 2 | Action 3 |
---|---|---|---|
State 1 | 0.25 | 0.5 | 0.75 |
State 2 | 0.1 | 0.4 | 0.6 |
State 3 | 0.8 | 0.2 | 0.9 |