Automate Web Page Clicks
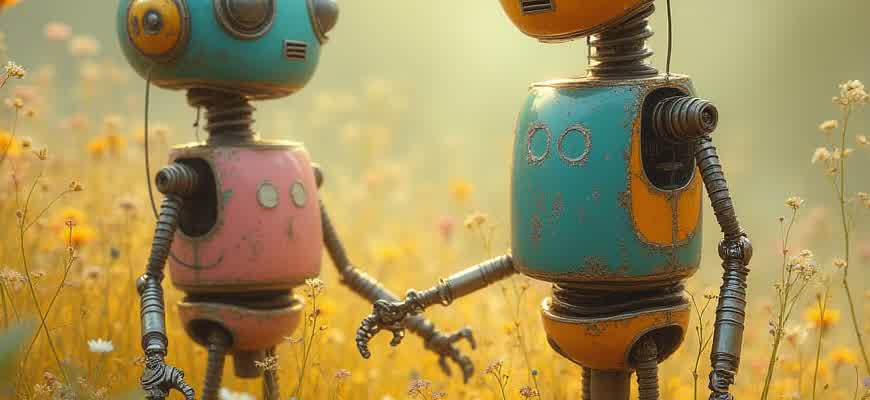
Automating the process of clicking on elements within a web page can significantly improve efficiency in repetitive tasks, such as form submissions, data extraction, or web testing. Through automated scripts, users can simulate human actions and execute clicks on various elements like buttons, links, or checkboxes. This method is particularly useful in scenarios where large-scale interaction with multiple web pages is necessary.
Important: Automation tools are essential for tasks such as load testing, performance analysis, and web scraping.
There are several tools and frameworks available to implement automation, with the most popular ones being Selenium and Puppeteer. These tools allow users to control web browsers and simulate clicks by interacting with page elements using selectors, such as IDs, class names, or XPath expressions.
- Use of selectors: Identifying the right element is crucial for effective automation.
- Managing dynamic content: Handling pages with changing content requires advanced scripting techniques.
- Handling delays: Automation should account for network or rendering delays to avoid errors in interaction.
For more complex scenarios, automated systems can be programmed to click multiple items in sequence, making it possible to interact with pages as if a human were navigating them. The key to success lies in accurately identifying each element and ensuring proper synchronization with the web page's behavior.
Framework | Language | Primary Use |
---|---|---|
Selenium | Java, Python, C#, Ruby | Browser automation for web testing |
Puppeteer | JavaScript | Headless browser automation for scraping and testing |
Understanding the Basics of Web Page Click Automation
Automating web page interactions has become an essential tool for various web scraping, testing, and business process automation tasks. One of the primary actions that can be automated is simulating clicks on buttons, links, and other interactive elements. This automation helps in reducing manual intervention and enhances the speed and accuracy of tasks like form submissions, testing website functionality, or interacting with dynamic web pages.
Click automation is generally achieved through scripting and browser automation tools, which allow for simulating human-like interactions with web elements. Tools like Selenium, Puppeteer, and Playwright are widely used for this purpose. They enable developers to create scripts that can locate and interact with specific page elements, performing clicks based on pre-defined conditions and actions.
Key Concepts in Click Automation
Several concepts and steps are fundamental to understanding how click automation works. Below are some of the critical components of the process:
- Element Identification: Locating the HTML elements (e.g., buttons, links, checkboxes) on the web page is the first step. This is typically done using selectors such as CSS selectors, XPath, or IDs.
- Action Triggers: Identifying the actions (click events) that should be triggered on these elements, which could include simulating a click, mouse hover, or even filling out forms.
- Browser Interaction: The automation tool interacts with a browser (Chrome, Firefox, etc.) to execute these actions as if a user were doing them manually.
Automation scripts often include conditional logic to handle dynamic content, such as waiting for elements to load or interact only when certain conditions are met. These tools typically simulate real user behavior as closely as possible, ensuring that web applications react as expected.
"Automation scripts simulate real-time user interactions by waiting for specific elements to load, clicking buttons, and capturing data, which significantly reduces human error."
Tools for Automating Clicks
Several tools are widely used for automating web page clicks:
- Selenium: One of the most popular tools for browser automation, Selenium provides bindings for multiple programming languages like Python, Java, and JavaScript.
- Puppeteer: Built for controlling headless browsers like Google Chrome, Puppeteer is particularly effective for scraping data and automating clicks on dynamic web pages.
- Playwright: Similar to Puppeteer, Playwright supports multiple browsers and offers advanced automation capabilities for modern web applications.
Example of Automation Flow
Below is a simple table that illustrates how an automation script typically works when simulating a click action:
Step | Action | Tool/Method |
---|---|---|
1 | Locate the button | CSS Selector, XPath |
2 | Wait for the button to be clickable | Explicit wait in Selenium |
3 | Click the button | Click method (e.g., `click()` in Selenium) |
4 | Verify the outcome | Assertions in test scripts |
Key Tools for Automating Clicks on Web Pages
When automating tasks on web pages, selecting the right tools is crucial to ensure seamless operation and accuracy. There are several types of software and frameworks that cater to different needs when it comes to simulating user interactions, such as clicking on elements like buttons, links, or other interactive components. These tools vary in complexity, ease of use, and customization options, making it important to choose one based on the specific requirements of your project.
Below is a list of some of the most popular tools for automating web page clicks, along with an overview of their capabilities. Each of these tools can help streamline repetitive tasks, conduct tests, or enhance user experience simulations.
Popular Tools for Automating Web Interactions
- Selenium – A widely used framework for automating browsers, Selenium supports various programming languages, including Java, Python, and JavaScript. It allows users to programmatically interact with a web page, including clicking elements, filling out forms, and navigating through pages.
- Playwright – A modern automation tool developed by Microsoft. Playwright offers fast, reliable browser automation and supports Chromium, WebKit, and Firefox. It is well-suited for cross-browser testing and automating complex interactions.
- Puppeteer – A Node.js library that provides a high-level API for controlling Chrome or Chromium. Puppeteer is particularly useful for web scraping and automating tasks like clicking, form submission, and even generating screenshots.
Key Features and Considerations
Tool | Programming Language | Best Use Case |
---|---|---|
Selenium | Java, Python, JavaScript, C# | Cross-browser testing and automating web interactions |
Playwright | JavaScript, Python, C# | Fast browser automation and cross-browser testing |
Puppeteer | JavaScript | Web scraping, headless browser interactions |
Note: While all of these tools are powerful, the choice between them often depends on the complexity of the tasks, your preferred programming language, and the browsers you need to test or automate.
How to Set Up Click Automation Scripts for Your Website
Automating clicks on your website can greatly improve efficiency, especially when handling repetitive tasks. This can be achieved using browser automation tools like Selenium or Puppeteer, which allow you to simulate user interactions like clicking buttons, filling out forms, and navigating pages. By setting up click automation, you can streamline user experience testing, conduct data scraping, or perform other actions that require interaction with the web elements.
In this guide, we'll walk you through setting up a simple automation script for handling clicks on your website. The process involves selecting the correct tools, writing scripts that target specific elements, and executing them for various purposes.
Step-by-Step Guide to Automating Clicks
- Select an Automation Tool: Choose a browser automation framework that fits your needs. Common tools include:
- Selenium
- Puppeteer
- Playwright
- Install Required Packages: Depending on your choice of tool, install the corresponding libraries. For example, with Node.js, you can install Puppeteer via npm:
npm install puppeteer
- Write the Click Automation Script: Write a script that simulates the clicks. A simple Puppeteer script to click a button might look like:
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch();
const page = await browser.newPage();
await page.goto('https://example.com');
await page.click('#myButton');
await browser.close();
})();
- Run the Automation Script: Execute your script and monitor the results. The script will automatically perform clicks on the specified elements, simulating a real user interaction.
Useful Tips and Recommendations
Tip | Explanation |
---|---|
Test Element Selectors | Ensure that the CSS selectors or XPath expressions are correct to accurately target the elements you want to interact with. |
Delay Between Clicks | Use delays between interactions to make the automation appear more natural, avoiding detection by anti-bot mechanisms. |
Headless Mode | Run the script in headless mode (without opening a browser window) to speed up execution. For Puppeteer, this is the default setting. |
Common Pitfalls in Web Page Click Automation and How to Avoid Them
Automating clicks on web pages can significantly improve efficiency, but it comes with its own set of challenges. Understanding these common issues is crucial for smooth automation and avoiding potential disruptions. In this guide, we’ll highlight the most frequent mistakes developers make and provide solutions to overcome them.
While automating interactions with web pages, it’s easy to overlook small details that can lead to issues like failed clicks or delayed actions. Below are the most common pitfalls in web automation and the best practices to prevent them.
1. Element Selection Failures
One of the most frequent problems is selecting the wrong elements on the page, either due to dynamic changes or incorrect locators. This results in clicks being directed to the wrong parts of the page or failing altogether.
Tip: Use robust and unique selectors such as CSS selectors or XPath expressions that remain consistent even when the page layout changes.
- Ensure that the element is visible and not hidden behind overlays.
- Check for dynamic IDs or class names that change every session.
- Use waits or retries to handle slow-loading elements.
2. Unreliable Wait Times
Automating clicks too soon before a page has fully loaded or an element has become interactive often leads to failure. Fixed delays (e.g., sleep() in code) can cause unnecessary waits or premature actions.
Tip: Implement dynamic waits that only proceed when an element is ready, such as WebDriver’s Explicit Waits.
- Use conditions like "element to be clickable" instead of arbitrary wait times.
- Implement "implicit waits" to automatically wait for elements to appear within a specified time frame.
3. Interaction with Dynamic Content
Many modern websites use JavaScript to dynamically load content. Automating clicks on such pages can be tricky, as elements may not be available immediately after the page loads.
Tip: Test the visibility and readiness of dynamic elements before interacting with them using tools like waitFor() or waitForElement().
Dynamic Element Type | Recommended Solution |
---|---|
AJAX-loaded content | Use waitForElement or similar functions to detect the element’s readiness. |
Pop-ups and Modals | Ensure the modal is completely loaded and visible before interacting. |
Integrating Click Automation with Google Analytics for Data Tracking
Automating user interactions with web pages is an effective strategy to enhance testing and data collection processes. One of the most powerful ways to leverage automation is by integrating it with analytics tools like Google Analytics. This combination allows for a more streamlined and accurate approach to tracking user behavior, particularly clicks and interactions. By automating clicks, businesses can generate large volumes of data, which can then be analyzed for insights into user engagement, conversion rates, and overall website performance.
Google Analytics provides a robust platform for tracking and analyzing user actions. When combined with automated click actions, it allows for detailed reports on how visitors interact with specific elements on the page. This integration enables businesses to track whether users are clicking on call-to-action buttons, navigation links, or other key elements, all without manual testing. By automating these interactions, you can collect data across a wide range of conditions and use it to make data-driven decisions.
How Click Automation Enhances Data Tracking
- Efficiency in Testing: Automated clicks simulate real user behavior, allowing for fast and repetitive testing of various page elements without human intervention.
- Improved Accuracy: Automation eliminates the possibility of human error in data collection, ensuring more consistent and reliable results over time.
- Increased Data Volume: By automating clicks, a larger volume of data can be collected in a shorter period, providing a more comprehensive view of user interactions.
Setting Up Click Tracking with Google Analytics
- Integrate Google Analytics with your website using the tracking code provided by Google.
- Set up event tracking within Google Analytics to monitor specific clicks, such as button presses or link activations.
- Use a click automation tool like Selenium or Puppeteer to simulate user interactions on the page, triggering the events you want to track.
- Verify that the event data is being captured in Google Analytics, ensuring that the click actions are being recorded as intended.
By automating clicks and integrating with Google Analytics, businesses can gather valuable insights into how users engage with their site, making it easier to optimize for better user experiences and higher conversion rates.
Example of Event Tracking Setup
Action | Event Category | Event Action | Event Label |
---|---|---|---|
Click on Sign Up Button | Button Clicks | Sign Up | Sign Up Page |
Click on Learn More Link | Link Clicks | Learn More | Product Page |
How to Personalize Click Patterns for Accurate User Simulation
When it comes to simulating user behavior on web pages, customizing click patterns can help replicate real-world interactions more closely. It is essential to account for various user habits, including their browsing speed, frequency of interactions, and the sequence of actions taken. By adjusting click patterns based on these factors, the simulation becomes more realistic and can yield better insights into user experience and performance metrics.
In this guide, we explore methods for refining click sequences by considering multiple user-specific factors and adapting patterns accordingly. This allows for precise analysis of how users might interact with a website's elements, from buttons and links to dropdowns and forms.
Factors Influencing Click Patterns
- Click Timing: Determine how quickly users click on elements after they load, as this can vary from one user to another.
- Frequency of Interaction: Consider how often a user clicks on certain elements, whether they visit a page once or multiple times.
- Click Sequence: Simulate logical order based on typical browsing patterns, such as reading first, then clicking.
- Area of Interest: Customize clicks based on which part of the page is most engaging for the user (e.g., main content, navigation bar).
Steps to Customize Click Behavior
- Identify User Types: Categorize users based on behaviors such as first-time visitors or frequent users, which will influence how they click.
- Define Interaction Patterns: Map out typical user flows, such as visiting a product page, adding to cart, and checking out.
- Adjust Timing and Speed: Modify the time delay between interactions to reflect natural user pacing.
- Test and Refine: Continuously monitor the accuracy of click patterns and adjust them based on feedback or testing results.
Example Custom Click Patterns
User Type | Click Pattern | Time Delay (Seconds) |
---|---|---|
First-time Visitor | Scroll, Click Navigation, Click Product | 3-5 |
Frequent User | Click Navigation, Direct Purchase | 1-2 |
Casual Visitor | Scroll, Click Links, Exit | 4-6 |
Remember, accurate click simulation depends on capturing real-world user behaviors. Testing different variations and iterating over time is essential to achieving the best results.
Testing and Debugging Click Automation Flows
When automating web page interactions, especially clicks, ensuring that the automated flows work seamlessly is essential. Testing these flows helps in identifying areas where automation might fail, whether due to UI changes or incorrect assumptions in the code. Comprehensive testing helps improve the robustness and reliability of automated scripts, ensuring that they mimic real-user behavior and handle edge cases effectively.
Debugging automated clicks can be challenging because of the dynamic nature of web pages. Often, issues arise from elements being hidden, not loaded, or interacting unexpectedly with other components. This requires a methodical approach to isolate the problem and adapt the automation flow accordingly. Using tools such as breakpoints and logging can be crucial in pinpointing where the flow breaks down.
Key Strategies for Effective Testing
- Verify element visibility and availability before interaction.
- Simulate real-user behaviors, such as waiting for elements to load or become interactive.
- Test across multiple browsers and devices to ensure consistent behavior.
Common Debugging Techniques
- Use browser developer tools to inspect elements and ensure proper identification in the automation script.
- Implement error handling to catch exceptions and retry actions when necessary.
- Log detailed information about each step of the automation process for easier tracking of failures.
Debugging with Logs and Screenshots
Logs and screenshots are invaluable tools for tracking down issues in automated flows. Logs help identify the exact point of failure, while screenshots capture the state of the web page when the issue occurred.
Example Table: Common Testing Issues
Issue | Solution |
---|---|
Element not found | Ensure the element is visible and loaded before interacting with it. Use explicit waits. |
Incorrect click position | Verify the target element is in the viewport and can be clicked. |
Unexpected page redirects | Use try-catch blocks to handle redirections and wait for the page to stabilize. |
Legal and Ethical Aspects of Automating Web Clicks
Automated interactions with websites, such as simulating user clicks, can present numerous challenges from both a legal and ethical standpoint. While these automation techniques are widely used in various industries, it is important to understand the rules and guidelines that govern their application to avoid potential risks. Automation can cross legal boundaries if it infringes on intellectual property or violates privacy rights, while ethically it may harm user experience and undermine fair practices on the web.
Before implementing click automation tools, it is essential to be aware of the legal restrictions in place, including data protection laws and terms of service agreements. Additionally, the ethical implications of such automation cannot be overlooked, especially regarding the fairness of competition and the preservation of privacy.
Legal Considerations
- Terms of Service Violation: Many websites explicitly prohibit automated access in their user agreements. Ignoring these rules can result in legal repercussions such as account termination or lawsuits.
- Copyright Infringement: Automated clicks that scrape or reproduce content from a website can breach copyright laws, leading to potential legal disputes.
- Non-compliance with Data Privacy Laws: Automation tools that collect personal data without user consent may violate laws like the GDPR or CCPA, carrying heavy fines.
Ethical Issues
"The use of automation should prioritize fairness, privacy, and the overall integrity of the web environment."
- Unfair Advantage: Using automated clicks to manipulate metrics or inflate traffic numbers can create an unfair competitive edge and distort market dynamics.
- Decreased User Experience: Excessive automation may overload web servers or reduce the effectiveness of services designed for human interaction.
- Privacy Violations: Automating the collection of sensitive user information without transparency or consent is an unethical practice that undermines trust.
Legal Guidelines Overview
Legal Aspect | Consequences |
---|---|
Violation of Terms of Service | Potential legal action and termination of access to the site. |
Data Privacy Breaches | Large fines and reputational damage for non-compliance with regulations. |
Copyright Violations | Legal disputes and potential loss of intellectual property rights. |