Qt Push Button Signals
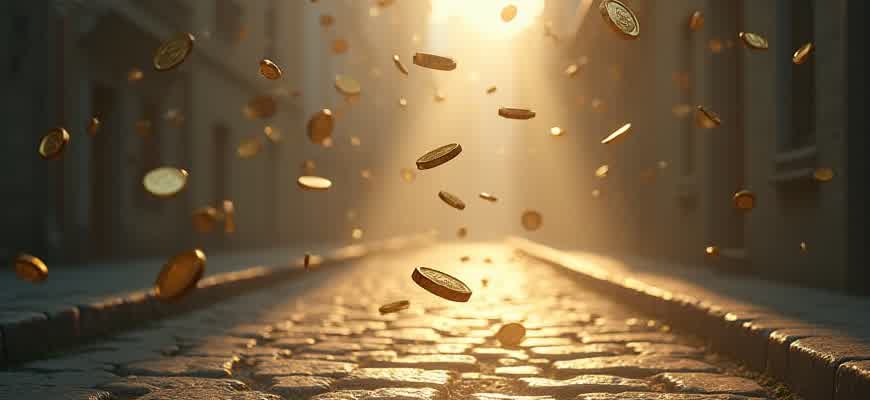
In Qt, Push Buttons are interactive widgets that allow users to trigger actions within an application. These buttons are commonly used in GUI development and can be connected to various signals that activate specific slots. The most common signal associated with a button is clicked(), which is emitted when the user clicks on the button. Understanding how to work with these signals is key to building dynamic applications that respond to user input.
Below is a list of some key signals related to push buttons in Qt:
- clicked() - Emitted when the button is clicked by the user.
- pressed() - Emitted when the button is pressed down.
- released() - Emitted when the button is released.
- toggled() - Emitted when a button's state changes, for toggle buttons.
Note: Each signal can be connected to a slot, which is a function that is executed in response to the signal being emitted. This enables reactive behavior in your application.
When working with these signals, developers can define custom slots to handle the events in various ways. For example, connecting the clicked() signal to a slot might trigger a function that performs an action, like updating a label or performing a calculation. The connection between a signal and a slot is achieved using the connect() method in Qt.
Signal | Slot | Action |
---|---|---|
clicked() | onButtonClicked() | Executes when the button is clicked |
pressed() | onButtonPressed() | Executes when the button is pressed |
Implementing Multiple Handlers for a Single Push Button Signal
In Qt, a signal emitted by a push button can be connected to multiple handlers. This allows the button to trigger several actions when pressed, providing flexibility in user interface design. By connecting multiple slots or functions to the same signal, developers can execute different operations in response to a single user interaction, without needing to duplicate code or create unnecessary UI elements.
To achieve this, Qt offers various methods for connecting multiple signal handlers, such as using the QObject::connect()
function or leveraging the QSignalMapper
for more complex scenarios. Each approach allows the developer to associate multiple functions or slots with the same signal, creating a dynamic response to the button press.
Steps to Connect Multiple Handlers
- Define the functions or slots that should handle the signal.
- Use
QObject::connect()
to bind multiple handlers to the signal of the push button. - Ensure that the signal is emitted, triggering all connected slots.
Example of Multiple Handlers
connect(button, &QPushButton::clicked, this, &MyClass::handlerOne);
connect(button, &QPushButton::clicked, this, &MyClass::handlerTwo);
Table of Options
Method | Description |
---|---|
QObject::connect() | Directly connects the signal to multiple slots or functions. |
QSignalMapper | Maps multiple signals to a single slot, useful for dynamically handling signals from several buttons. |
Note: Connecting multiple handlers is a common approach when different actions need to be executed in response to a single event, such as updating the UI and performing background tasks simultaneously.
Best Practices for Connecting Signals and Slots in Qt
When working with Qt's signal-slot mechanism, it's important to follow best practices to ensure clean, efficient, and maintainable code. Signals and slots provide a flexible way to handle event-driven programming, but improper connections can lead to memory leaks, difficult-to-maintain code, or unexpected behavior. This guide explores the essential practices for properly handling these connections in Qt applications.
Here are some tips and guidelines for effectively using signals and slots in Qt:
Use Explicit Connections for Clarity
Explicit connections are often preferable as they make the code easier to read and maintain. They also allow you to check whether a connection has been successfully made. By using the QObject::connect
function directly, you can make the connections more transparent and avoid ambiguity that may arise from implicit connections.
Important: Avoid implicit connections unless absolutely necessary. They can introduce side effects that are difficult to debug, especially in larger applications.
Choose the Right Connection Type
In Qt, there are different connection types depending on the thread context of the sender and receiver. Make sure to choose the correct type based on whether you are working with different threads or single-threaded applications.
- AutoConnection: Default, most common and suitable for single-threaded apps.
- DirectConnection: Directly invokes the slot in the sender's thread.
- QueuedConnection: Slot is invoked in the receiver's thread, suitable for multi-threaded apps.
Avoid Connecting to Overloaded Slots
Overloading slots in Qt can lead to unintended behavior, especially if you expect the wrong version of a slot to be called. Always ensure that the signature of the slot matches the signal exactly, or use specific connections to avoid ambiguity.
Consider Memory Management
Memory management is crucial when connecting signals to slots, especially if objects are destroyed unexpectedly. Be careful with the lifetime of the connected objects to prevent accessing destroyed instances.
Important: Use QObject::destroyed
signal to automatically disconnect slots when the object is deleted.
Examples of Signal-Slot Connections
Here is an example of how to properly connect a push button signal to a slot in a Qt application:
QPushButton* button = new QPushButton("Click Me");
QObject::connect(button, &QPushButton::clicked, this, &MyClass::onButtonClicked);
Table of Connection Types
Connection Type | Use Case | Threading Behavior |
---|---|---|
AutoConnection | Most common | Determined by context |
DirectConnection | When sender and receiver are in the same thread | Invokes slot in sender's thread |
QueuedConnection | When sender and receiver are in different threads | Invokes slot in receiver's thread |
By following these best practices, you can ensure that your signal-slot connections are reliable, efficient, and safe, leading to more maintainable and effective Qt applications.
Debugging Push Button Signal Issues in Qt Applications
When working with Qt, the signal-slot mechanism is a core feature for handling user interactions like button clicks. However, there can be various issues when connecting signals from buttons to corresponding slots. Understanding and debugging these issues can save time and help ensure smooth interaction within your application.
Common problems often arise from incorrect signal-slot connections, signal emission issues, or even problems with the button's state. To identify and resolve these problems, a structured debugging approach is required. Below are some effective methods for troubleshooting signal problems in Qt buttons.
Steps to Debug Push Button Signal Issues
- Verify Signal-Slot Connections: Ensure the signal is properly connected to the appropriate slot using
QObject::connect()
. Mistakes in the function signature or object references often lead to missed signals. - Check Button State: Verify if the button is enabled, visible, and properly initialized. Disabled buttons do not emit signals.
- Use Qt's Debugging Tools: Utilize Qt's built-in
qDebug()
to print the signal emission and slot invocation.
Common Problems and Solutions
Problem | Solution |
---|---|
Signal Not Emitting | Ensure that the button is properly initialized and connected to the correct slot. Check if the button is enabled. |
Slot Not Responding | Check if the slot function signature matches the expected signal's signature. Ensure that the object is valid and connected correctly. |
Multiple Signals Not Connecting | Ensure that the connect statement is not being overridden or disconnected unexpectedly. Use Qt::UniqueConnection to prevent multiple connections. |
Tip: Use Qt’s QObject::dumpObjectInfo()
to inspect the connections between signals and slots. This can help identify any mismatches or unconnected signals.
Customizing Push Button Signals for Complex UI Interactions
When designing advanced user interfaces in Qt, one of the most important aspects is handling user input effectively. Push buttons are commonly used elements in UI design, and while they may seem simple, they can be customized to trigger complex interactions. By refining signal handling, developers can ensure that the application responds to user actions in sophisticated ways, providing a smoother and more intuitive experience.
Customizing signals allows developers to bind buttons to specific tasks, manipulate data, or trigger other events that are necessary for the interaction flow. Qt’s signal and slot mechanism provides the flexibility needed to extend default behavior. The ability to create custom signals, or even modify how existing ones behave, opens up numerous possibilities for interactive applications.
Advanced Signal Customization Techniques
There are several methods to enhance push button signals, making them more suitable for complex workflows:
- Connecting multiple actions to a single button.
- Creating conditional triggers based on specific user inputs.
- Triggering different actions depending on the button state (pressed, released, etc.).
Example Customization: Conditional Actions
By using conditional statements inside the connected slot, a single button can perform different tasks under various circumstances:
- Check the application state before executing a task.
- Trigger an animation or change button appearance after the first action.
- Handle special cases such as disabling the button after one press or changing its function dynamically.
Important: It’s essential to ensure that your signals are connected properly and that the slot behavior is synchronized with the UI context to prevent any UI lags or unexpected behavior.
Signal Customization Example Table
Button Action | Signal Type | Result |
---|---|---|
Button Pressed | clicked() | Executes an action immediately. |
Double Click | doubleClicked() | Triggers a complex dialog or a new window. |
Long Press | longPressed() | Changes button color or performs a background task. |