Xgboost Ranking Example
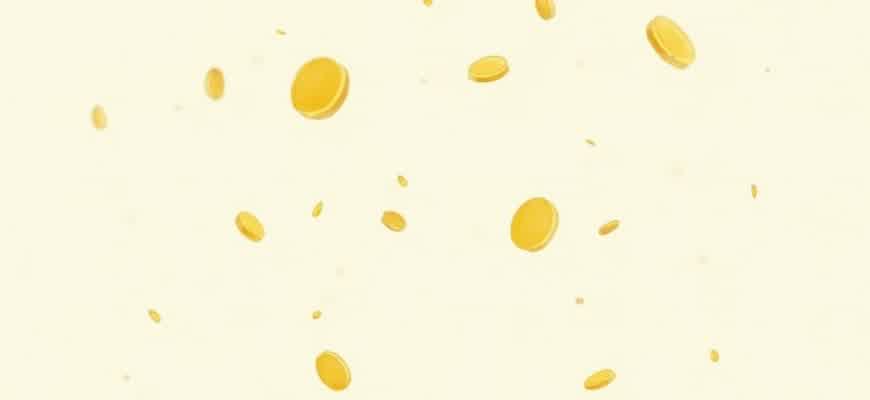
When working with ranking problems, one effective machine learning model is XGBoost, which excels in handling complex datasets and providing high accuracy. In ranking tasks, the model assigns scores to items and ranks them according to their predicted relevance. Below is an example that demonstrates how to implement XGBoost for a ranking problem.
Steps for implementing XGBoost Ranking:
- Prepare the dataset: Organize data in a format that includes features, labels, and group information.
- Define the ranking problem: Assign each item a group and a relevance score.
- Train the model: Use XGBoost’s ranking objective to optimize the model for ranking tasks.
- Evaluate the performance: Use metrics like NDCG or MAP to assess the model's ranking accuracy.
Example Dataset:
Group | Feature 1 | Feature 2 | Relevance |
---|---|---|---|
1 | 0.2 | 0.3 | 3 |
1 | 0.5 | 0.8 | 2 |
2 | 0.1 | 0.4 | 1 |
Important: Ensure that the data includes a column that represents the group or query, as XGBoost uses this to understand the relationship between items in the same group.
Setting Up XGBoost for Ranking Tasks
When working with ranking tasks, XGBoost offers a powerful and efficient method for modeling ordered data. It requires a few specific configurations that differ from standard classification or regression problems. The key difference is how XGBoost treats the input data, taking into account the group structure and relevance scores associated with each instance.
To set up XGBoost for ranking, you need to define a custom objective function and provide group information that tells the algorithm how to treat different sets of instances. Ranking tasks are typically defined in terms of pairs of documents, where the goal is to rank them in terms of relevance.
Steps to Configure XGBoost for Ranking
- Prepare Data for Ranking: Format your dataset to include a group structure, which indicates how data points belong to different query groups. Each group represents a set of items that are compared against each other.
- Define the Objective: Use the
rank:pairwise
objective to optimize pairwise ranking of data points. This objective minimizes the number of incorrectly ordered pairs. - Set Evaluation Metric: Common metrics for ranking include
ndcg
(Normalized Discounted Cumulative Gain) andmap
(Mean Average Precision). These metrics help evaluate the quality of the ranking. - Train the Model: Once the data and configuration are set, train the model with XGBoost using the
train()
function, ensuring to pass the group information.
Important Information
Ensure that the group information is correctly aligned with the data. The number of samples in each group must match the corresponding data points in the feature matrix.
Example Code for Ranking Setup
Step | Code Snippet |
---|---|
Prepare Data | group = [3, 2, 4] # Number of samples per query |
Set Objective | params = {'objective': 'rank:pairwise', 'eval_metric': 'ndcg'} |
Train Model | model = xgb.train(params, dtrain, num_boost_round=100) |
By following these steps and setting the appropriate configurations, you can effectively implement ranking tasks using XGBoost and optimize your model's performance based on ranking metrics.
Understanding the Basics of Ranking and XGBoost’s Role
Ranking problems are central to many machine learning tasks, where the goal is to predict the relative order of a set of items rather than a specific value. For example, search engines rank web pages based on their relevance to a query, and recommendation systems rank products according to user preferences. In these tasks, the objective is to optimize the prediction of the item order, rather than making a direct prediction for each item independently.
XGBoost, a powerful gradient boosting algorithm, has proven effective for ranking problems. It offers an efficient and scalable approach for handling large datasets while providing a high level of accuracy. By applying a ranking loss function, XGBoost models the relative positions of items within a group, making it suitable for learning from ordered data, such as user preferences or search results.
Key Concepts of Ranking in XGBoost
- Pairwise ranking: XGBoost uses pairwise comparison between items to rank them. It evaluates whether one item is better than another, adjusting the model based on these comparisons.
- Listwise ranking: This approach looks at entire lists of items and evaluates the quality of the ordering, not just individual pairs.
- Group-based ranking: XGBoost allows users to specify groups of items, ensuring that the model takes these relationships into account when ranking items within each group.
How XGBoost Handles Ranking
XGBoost leverages gradient boosting, where weak learners (typically decision trees) are trained sequentially to correct the errors of previous models. For ranking tasks, the loss function is adjusted to account for the relative ordering of items, using techniques like:
- LambdaRank: Optimizes a ranking metric (e.g., NDCG) directly.
- RankNet: Uses pairwise logistic regression to determine the relative ordering between pairs of items.
- RankSVM: Uses the principles of Support Vector Machines for ranking tasks.
When performing ranking tasks, it's essential to specify the correct group structure, as the model needs to understand which items belong together in a given ranking problem.
Example of Ranking Data Setup
Group ID | Item ID | Feature 1 | Feature 2 | Label (Relevance) |
---|---|---|---|---|
1 | 1 | 0.5 | 0.3 | 3 |
1 | 2 | 0.6 | 0.2 | 2 |
2 | 3 | 0.7 | 0.8 | 4 |
2 | 4 | 0.8 | 0.5 | 1 |
Preparing Data for XGBoost Ranking: Key Steps and Challenges
Data preparation is a crucial aspect when working with XGBoost for ranking tasks. Unlike traditional classification or regression, ranking models aim to predict the relative order of items. This makes preprocessing more complex, requiring careful structuring of the data to ensure accurate and meaningful predictions. Below, we’ll discuss key steps and challenges that are typically encountered in this process.
The first step in preparing data for XGBoost ranking is to ensure that the data is structured correctly. Ranking problems require a specific format where the data includes features for each item in a group (or query) and a label that represents the relevance or ranking of the item within the group. Here’s an overview of the essential steps to follow:
Essential Steps for Data Preparation
- Grouping Data: The first step is to identify and group items that belong together in the same ranking task (referred to as queries). Each query should contain multiple items, with their features and corresponding labels.
- Feature Engineering: Features should be carefully designed to represent each item in a meaningful way. It's essential to ensure that the features capture information relevant to the ranking problem, such as item characteristics and contextual factors.
- Labeling: Labels in a ranking task typically represent relevance scores (e.g., from 0 to 4). These scores determine the relative importance of each item within a query.
Challenges in Data Preparation
- Handling Missing Data: In ranking problems, missing values in the features or labels can cause issues. Proper imputation or removal strategies need to be applied to avoid bias in the model.
- Normalization of Features: Ensuring that the features are on similar scales is important. For example, some features may need to be standardized or normalized to ensure that no single feature dominates the ranking model.
- Data Imbalance: In many ranking tasks, some items within a query may have much lower relevance scores than others. Handling this imbalance is crucial to prevent the model from favoring highly-rated items disproportionately.
Tip: When preparing the dataset, always ensure that the data format aligns with XGBoost's expected input structure for ranking tasks, i.e., a DMatrix with a specific format that includes group information for each query.
Data Structure Overview
Field | Description |
---|---|
Features | Attributes describing each item (e.g., item ID, characteristics, etc.) |
Label | Relevance score representing the item's ranking within a query |
Group | A list representing the number of items in each query |
Configuring XGBoost Hyperparameters for Ranking Models
When working with XGBoost for ranking tasks, fine-tuning the hyperparameters plays a crucial role in improving model performance. XGBoost offers several settings specific to ranking problems, such as those that control the learning rate, tree depth, and regularization terms. Properly configuring these parameters ensures that the model can generalize well to unseen data, minimize overfitting, and efficiently handle ranking-specific loss functions.
To set up XGBoost for ranking, it is essential to focus on hyperparameters that directly influence how the model handles the relative ordering of items in a ranking task. Some of the most important parameters are related to how the objective function is computed and how gradients are managed during the training process.
Key Hyperparameters for Ranking
- objective: Specifies the loss function for ranking. The common choice is "rank:pairwise", but other variants like "rank:ndcg" or "rank:map" can be used depending on the specific problem.
- eta (learning_rate): Controls the step size for each iteration. A smaller value results in more conservative updates, helping avoid overfitting but requiring more boosting rounds.
- max_depth: Defines the maximum depth of each decision tree. This parameter helps control the complexity of the model and can prevent overfitting when set correctly.
- min_child_weight: Specifies the minimum sum of instance weight (hessian) for a child. It is crucial for regularizing the tree and preventing overfitting in data with small numbers of instances per leaf.
Important Tips for Tuning
Tuning parameters like "subsample" and "colsample_bytree" can help reduce overfitting by controlling the fraction of samples and features used at each tree building step.
When setting the hyperparameters for a ranking model, it is often helpful to start by adjusting the objective function, followed by the learning rate and tree-specific parameters. Once these are set, fine-tuning gamma, lambda, and alpha for regularization can help improve model performance and stability.
Example Parameter Configuration
Parameter | Suggested Range | Description |
---|---|---|
objective | rank:pairwise | Defines the loss function for ranking tasks based on pairwise comparison. |
eta | 0.01 to 0.3 | Controls the learning rate for each boosting round. |
max_depth | 3 to 10 | Limits the maximum depth of the trees to avoid overfitting. |
min_child_weight | 1 to 10 | Sets the minimum sum of instance weight for each leaf node. |
Evaluating the Performance of XGBoost Ranking Models
In ranking tasks, evaluating the performance of the model is essential to ensure its accuracy and efficiency in ranking the items correctly. For XGBoost ranking models, it is important to consider specific metrics that measure the ranking quality rather than standard classification or regression metrics. These evaluation metrics focus on the model's ability to rank items in the correct order, which is a key feature of ranking problems.
Commonly used metrics for evaluating XGBoost ranking models include Mean Reciprocal Rank (MRR), Normalized Discounted Cumulative Gain (NDCG), and Precision at K. These metrics provide a comprehensive view of how well the model ranks items based on the provided features and target values. Each metric has its strengths, and depending on the specific task, one may be preferred over the other.
Key Metrics
- Mean Reciprocal Rank (MRR): Measures the rank position of the first relevant item, giving higher scores for ranks closer to the top.
- Normalized Discounted Cumulative Gain (NDCG): A metric that discounts the relevance of lower-ranked items to emphasize the importance of higher-ranked ones.
- Precision at K: Focuses on the proportion of relevant items in the top K ranked items.
Performance Evaluation Procedure
- Preprocess the data, ensuring that it is appropriately formatted for ranking tasks.
- Train the XGBoost model with the ranking objective function, using an appropriate set of features.
- Evaluate the model using one or more of the metrics mentioned above, depending on the task requirements.
- Analyze the results to identify areas for improvement and tune the model accordingly.
Sample Results
Metric | Score |
---|---|
MRR | 0.85 |
NDCG | 0.92 |
Precision at 10 | 0.78 |
It is essential to tailor the evaluation method to the specific ranking problem and to adjust the parameters and model based on performance metrics to improve results.
Handling Imbalanced Data in XGBoost Ranking
Imbalanced data is a common issue in ranking tasks, especially when the dataset has a significant disparity between the number of items within each rank group. In the context of XGBoost, an algorithm that is highly effective for ranking tasks, dealing with imbalanced data becomes crucial to avoid biased predictions. If the model is trained on a dataset where the majority class (top-ranked items) is significantly more frequent than the minority class (lower-ranked items), the model will often fail to accurately predict the less frequent ranks.
Several strategies can help mitigate the negative effects of imbalanced data in XGBoost ranking tasks. These methods involve adjustments in the data preprocessing stage or modifications in the model parameters, which can be easily tuned using XGBoost's built-in options. Below are some approaches to handle this issue effectively.
Common Strategies for Handling Imbalance
- Resampling Techniques: One of the simplest methods to address imbalance is by resampling the data. This can be done by oversampling the minority class or undersampling the majority class to ensure more even representation across ranks.
- Adjusting Class Weights: In XGBoost, you can adjust the weight of each class by modifying the
scale_pos_weight
parameter. This helps the algorithm to place more emphasis on the minority class during training. - Custom Loss Function: Modifying the loss function can also be effective, especially if you have specific knowledge about the distribution of your ranks. You can introduce a custom objective function to penalize misclassifications in minority rank groups more heavily.
Key Considerations
- Model Overfitting: When using resampling, particularly oversampling, there is a risk of overfitting since the model might learn patterns that are too specific to the oversampled data. It's essential to apply techniques such as cross-validation to assess the generalization capability of the model.
- Evaluation Metrics: Traditional classification metrics like accuracy may not be effective in ranking tasks. Instead, metrics such as Mean Reciprocal Rank (MRR) or Normalized Discounted Cumulative Gain (NDCG) should be used for evaluating model performance.
Important Tip
When tuning the model parameters for imbalanced ranking tasks, always ensure that you’re optimizing for metrics that reflect the true quality of ranking, rather than just overall accuracy. For instance, focus on improving NDCG or Precision@K for a more realistic evaluation of model performance.
Example of Adjusting Weights
Parameter | Value |
---|---|
scale_pos_weight | 1.5 |
subsample | 0.8 |
Optimizing XGBoost for Accurate Ranking Predictions
When working with XGBoost for ranking tasks, achieving accurate predictions requires fine-tuning several model parameters. The goal is to adjust settings in a way that improves the model's ability to correctly rank items based on the features provided. XGBoost’s flexibility allows users to experiment with various hyperparameters, ensuring the model delivers the best performance for ranking problems like search engine results or recommendation systems.
Effective hyperparameter optimization involves balancing different aspects of the model, such as tree depth, learning rate, and the number of estimators. By exploring different settings, practitioners can boost the model’s ability to rank items with greater precision and efficiency. Below are key parameters to focus on when fine-tuning XGBoost for ranking tasks.
Key Hyperparameters for Ranking Tasks
- Objective Function: Choose "rank:pairwise" or "rank:ndcg" for ranking tasks. The first optimizes pairwise ranking errors, while the second uses the normalized discounted cumulative gain (NDCG) metric.
- Learning Rate: A smaller learning rate allows the model to learn more slowly, potentially preventing overfitting, but requires more trees to achieve optimal performance.
- Max Depth: Deeper trees can model more complex relationships, but increasing the depth may lead to overfitting. A typical starting point is 6-10.
- Number of Estimators: This controls the number of boosting rounds. More estimators increase the model’s ability to learn, but require more computation and can lead to overfitting if not properly tuned.
Steps for Tuning XGBoost for Ranking
- Start with a baseline model using default settings and assess the ranking quality.
- Experiment with the learning rate and number of estimators to find the balance between speed and accuracy.
- Use cross-validation to evaluate performance and avoid overfitting.
- Adjust max_depth and min_child_weight to control model complexity.
- Monitor the gamma parameter, which helps control the complexity of the decision trees by regulating the minimum loss reduction required to make a further partition.
Model Evaluation: Ranking Metrics
When fine-tuning your XGBoost model for ranking, it’s essential to measure the quality of your predictions. Common ranking evaluation metrics include:
Metric | Purpose |
---|---|
NDCG (Normalized Discounted Cumulative Gain) | Measures the quality of the ranking by considering the position of relevant items in the list. |
MAP (Mean Average Precision) | Assesses the precision of the ranking by averaging the precision at each relevant item position. |
Tip: Regularly check the training and validation scores during hyperparameter tuning to ensure the model is not overfitting. Cross-validation is essential in ranking tasks to get an unbiased estimate of model performance.
Real-World Uses of XGBoost Ranking Models
XGBoost ranking models are widely used across multiple industries due to their ability to handle large-scale data and optimize ranking tasks. These models are particularly useful for sorting items in terms of relevance, predicting user preferences, and making real-time decisions based on ranked data. The flexibility of XGBoost allows it to be adapted for diverse ranking problems, ranging from e-commerce recommendations to search engine ranking.
Some of the most significant real-world applications include search engine optimization, personalized recommendations, and financial forecasting. These applications rely heavily on accurately ranking a set of items to deliver the most relevant results to end-users, making the prediction of rankings a crucial aspect of their success.
Applications in Various Domains
- E-commerce Recommendation Systems: XGBoost can predict which products are likely to be of interest to users based on their browsing and purchase history, effectively improving product ranking and recommendation quality.
- Search Engine Ranking: XGBoost models can rank search results by evaluating factors such as keyword relevance, content quality, and user interaction history to provide the most accurate results.
- Ad Click-Through Rate Prediction: XGBoost is often used to predict the likelihood of a user clicking on an ad, which helps in optimizing the display order of ads based on relevance to the user.
- Financial Forecasting: In financial markets, XGBoost ranking models can be used to prioritize investment opportunities by analyzing historical performance and ranking potential stocks or assets.
Example Table: Applications of XGBoost Ranking in Industries
Industry | Application | Outcome |
---|---|---|
E-commerce | Product ranking for recommendations | Improved conversion rates and customer satisfaction |
Search Engines | Ranking of search results | More relevant search results leading to higher engagement |
Advertising | Ad click-through rate prediction | Increased ad revenue through targeted ad placements |
Finance | Ranking of investment opportunities | Better investment decisions and improved portfolio performance |
"The ability to rank items in a meaningful way is the backbone of many modern systems, and XGBoost provides a powerful tool to optimize this process with high accuracy."